In this tutorial, you’ll learn how to use Python to convert an int to a binary, meaning converting an integer to a binary string.
You’ll learn a brief overview of this conversion and how binary strings are represented in computers. Then, you’ll learn how to use four different methods to use Python to convert int to binary. These include, the bin()
function, string formatting, f-strings, the format()
function, as well as naive implementation without the use of any functions.
The Quick Answer: Use the format() Function
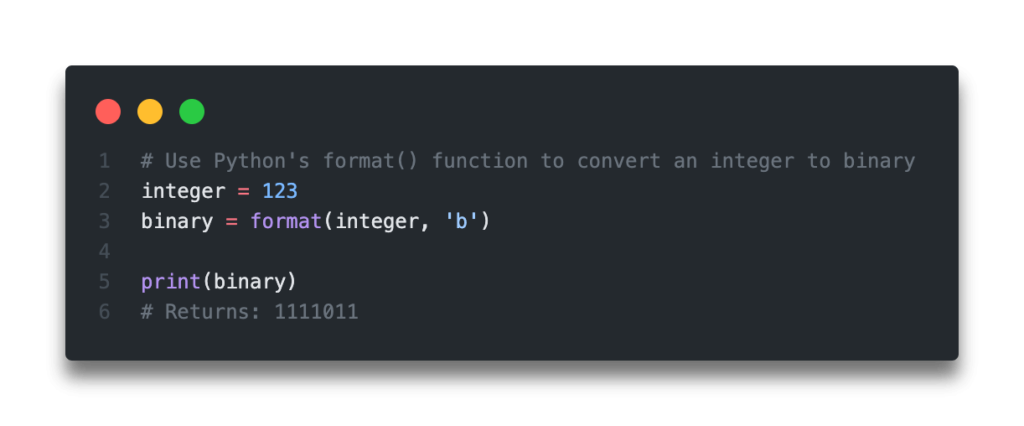
Table of Contents
What are Binary Strings for Integers?
The common integer system that we’re used to, the decimal system, uses a base of ten, meaning that it has ten different symbols. These symbols are the numbers from 0 through to 9, which allow us to make all combinations of numbers that we’re familiar with.
Binary strings, on the other hand, use a base of two, meaning that they only have two numbers to express different numbers. These numbers are either 0 or 1. While the binary number system has been in use in different ancient civilizations (such as Egypt and India), it is used extensively in electronics and computer system in modern times.
In the next sections, you’ll learn how to use Python to convert an integer to a binary using the bin()
function.
Want to learn how to get a file’s extension in Python? This tutorial will teach you how to use the os and pathlib libraries to do just that!
Use Python bin to Convert Int to Binary
The Python bin()
function is short for binary and allows us to convert an integer to a binary string, which is prefixed by '0b'
. In later section, you’ll learn how to convert the integer using Python without the prefix.
Let’s take a look at how we can turn a positive integer into a binary string using Python:
# Convert an integer to a binary string using Python bin()
positive = 123
binary = bin(positive)
print(binary)
Returns: '0b1111011'
We can see that a binary string with the '0b'
prefix has been returned.
Let’s check the type of the statement that’s been returned, using the built-in type()
function:
# Checking the type of our binary string
positive = 123
binary = bin(positive)
print(type(binary))
# Returns:
# <class 'str'>
We can see here that the function returned a string, as expected.
Now let’s see what happens when we pass in a negative integer and try to use Python to convert it to binary string:
# Convert an integer to a binary string using Python bin()
negative = -123
binary = bin(negative)
print(binary)
Returns: '-0b1111011'
We can see that there’s also a '-'
prefix to our string, letting us know that the number is a negative value.
In the next section, you’ll learn how to use Python string formatting to convert an int to a binary string.
Want to learn how to use the Python zip()
function to iterate over two lists? This tutorial teaches you exactly what the zip()
function does and shows you some creative ways to use the function.
Use Python String Formatting to Convert Int to Binary
If you’re wanting to convert a Python integer to a binary string without the '0b'
prefix, you can use string formatting.
Python string formatting allows us to define different format types for passing in values. In this case, we’ll pass in the format code of '{0:b}'
, which allows us to convert an integer to binary.
Let’s see how we can pass in a few integer values, both positive and negative, and convert them to binary string using string formatting:
# Convert an integer to a binary string using Python string formatting
positive = 123
negative = -123
positive_binary = '{0:b}'.format(positive)
negative_binary = '{0:b}'.format(negative)
print(f'{positive_binary=}')
print(f'{negative_binary=}')
# Returns:
# positive_binary='1111011'
# negative_binary='-1111011'
We can see here that this method returns the same strings, without the '0b'
prefix.
In the next section, you’ll learn Python f-strings to convert an int to a binary string.
Want to learn more about Python for-loops? Check out my in-depth tutorial that takes your from beginner to advanced for-loops user! Want to watch a video instead? Check out my YouTube tutorial here.
Use Python f-strings to Convert Int to Binary
Python f-strings allow us to make string formatting a little bit more intuitive. They also allow us to apply formatting to our strings in similar ways to traditional string formatting.
As a quick refresher on Python f-strings, they’re strings introduced in Python versions 3.6 and above, and are created by prefixing either a 'f'
or 'F'
to the string.
Let’s see how we can convert an integer to a binary string using Python f-strings. We’ll try this for the same positive and negative integers as above:
# Convert an integer to a binary string using Python f-strings
positive = 123
negative = -123
positive_binary = f'{positive:b}'
negative_binary = f'{negative:b}'
print(f'{positive_binary=}')
print(f'{negative_binary=}')
# Returns:
# positive_binary='1111011'
# negative_binary='-1111011'
We can see here that the same values are returned. Python f-strings may not work in all versions of Python, but they are intuitive and easy to understand.
In the next section, you’ll learn how to use the Python format()
function to convert an int to a binary string.
Want to learn more about Python f-strings? Check out my in-depth tutorial, which includes a step-by-step video to master Python f-strings!
Use Python format to Convert Int to Binary
Another way to convert a Python integer to a binary string is to use the built-in format()
function. The format()
function takes value and a format spec as its arguments.
Because of this, we can pass in a value (in this case, an integer) and a format spec (in this case “b”), to specify that we want to return a binary string.
Let’s see how we can accomplish this using Python:
# Convert an integer to a binary string using Python format()
positive = 123
negative = -123
positive_binary = format(positive, 'b')
negative_binary = format(negative, 'b')
print(positive_binary)
print(negative_binary)
# Returns:
# positive_binary='1111011'
# negative_binary='-1111011'
This is also a very readable way in which we can convert Python integers to string. The function makes it clear that we’re converting a value to something else, even specifying a type.
In the final section, you’ll learn how to convert an int to a binary string from scratch.
Want to learn how to calculate and use the natural logarithm in Python. Check out my tutorial here, which will teach you everything you need to know about how to calculate it in Python.
Convert an Int to Binary in Python without a Function
In this final section, you’ll learn how to convert how to create a naive method to convert a Python integer to a string. You’ll actually create a custom function that does this, but be able to understand how the conversion works.
Practically speaking, this is not something you’d really need to do, but it can be a helpful task to understand for programming interviews.
# Convert an integer to a binary string using a custom function
def int_to_binary(integer):
binary_string = ''
while(integer > 0):
digit = integer % 2
binary_string += str(digit)
integer = integer // 2
binary_string = binary_string[::-1]
return binary_string
print(int_to_binary(123))
# Returns:
# 1111011
Check out some other Python tutorials on datagy, including our complete guide to styling Pandas and our comprehensive overview of Pivot Tables in Pandas!
Conclusion
In this post, you learned how to use Python to convert int to binary, meaning how to convert integer values to binary strings. You learned how to do this using a number of different methods, including using the Python bin()
function, string formatting, f-strings, the format()
function, and without any functions at all.
If you want to learn more about the Python bin()
function, check out the official documentation here. To learn more about the Python format()
function, you can find the official documentation here.