In this tutorial, you’ll learn how to use Python to get the index of the max item in a list. You’ll learn how to find the index of the max item in a Python list, both if duplicates exist and if duplicates don’t exist.
You’ll learn how to do this with for loops, the max()
and index()
functions, the enumerate()
function, and, finally, using the popular data science library, numpy
.
Knowing how to work with lists is an important skill for anyone learning Python. Python lists are incredibly common and intuitive data structures in Python. Knowing how to find where the max (or, really, min) values exist in a list is an important skill.
The Quick Answer: Use index()
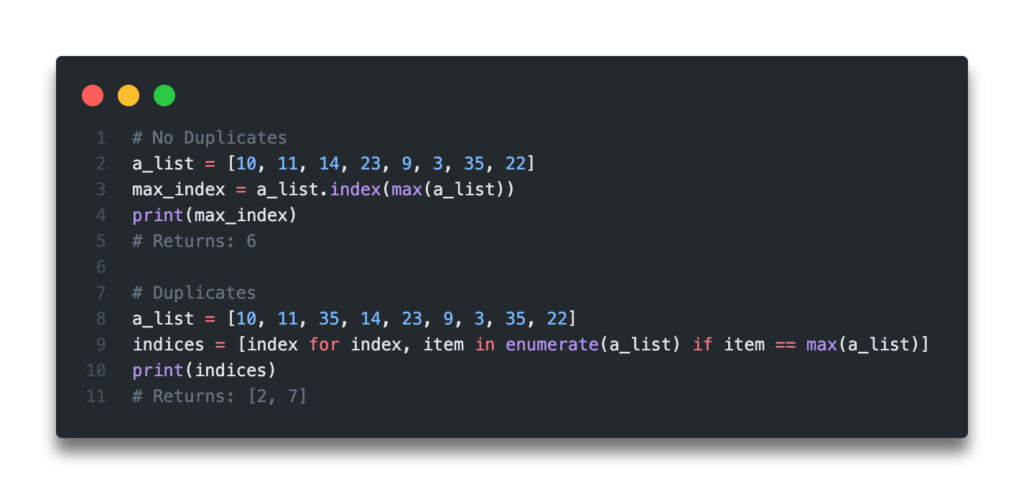
Table of Contents
Find the Max Value in a Python List
Before we dive into how to find the index of the max value in a list, lets begin by learning how to simply find the max value of an item in a list.
The Python max()
function takes one or more iterable objects as its parameters and returns the largest item in that object (official documentation). When we pass in a list, the function returns the maximum value in that list.
Let’s see what this looks like in practice:
# Get Max Value from a List
a_list = [10, 11, 14, 23, 9, 3, 35, 22]
print(max(a_list))
# Returns 35
We can see that when we pass in our list into the Python max()
function, that the value 35
is returned.
We’ll be using this function to evaluate against throughout the rest of the tutorial.
In the next section, you’ll learn how to return the index of the maximum value if no duplicates exist or you only need the first item.
Want to learn how to use the Python zip()
function to iterate over two lists? This tutorial teaches you exactly what the zip()
function does and shows you some creative ways to use the function.
Find Index of Max Item in Python List using index
Now that you know how to identify what the max value in a Python list is, we can use this along with the .index()
method to find the index of the max value.
One thing to note about this is that this method will return the first instance of the maximum value in that iterable object. If the object contains duplicate items, only the first will be returned. All the other methods shown below will return all of the indices, if duplicates exist.
Let’s see how we can use the .index()
method to return the index of the max value:
# Get Index of Max Value from a List
a_list = [10, 11, 14, 23, 9, 3, 35, 22]
max_value = max(a_list)
max_index = a_list.index(max_value)
print(max_index)
# Returns: 6
# You could also write:
# max_index = a_list.index(max(a_list))
What we’ve done here is applied the .index()
method to our list a_list
. The method takes an argument that indicates what item the method should find. The method will return the first (and, in this case, the only) index position of that value.
In the next section, you’ll learn how to use a Python for-loop to find the index or indices of the max item in a Python list.
Want to learn more about calculating the square root in Python? Check out my tutorial here, which will teach you different ways of calculating the square root, both without Python functions and with the help of functions.
Find Index of Max Item in Python List with a For Loop
In the method above, you learned how to use the Python .index()
method to return the first index of the maximum value in a list.
The main limitation of that approach was that it only ever returned the first index it finds. This can be a significant limitation if you know that duplicate values exist in a list. Because of this, this section will teach you how to use a Python for loop to find the indices of the highest value in a Python list.
Let’s see how this works in Python:
# Get Indices of the Max Value from a List using a for loop
a_list = [10, 11, 35, 14, 23, 9, 3, 35, 22]
indices = []
max_value = max(a_list)
for i in range(len(a_list)):
if a_list[i] == max_value:
indices.append(i)
print(indices)
# Returns: [2, 7]
What we’ve done here is:
- Initialized two lists: our list
a_list
which contains our values, and an empty list that we’ll use to store the indices. - We created a variable that stores the max value
- We then looped over each item in the list to see if it matches the item
- If it does, we return its index
In the next section, you’ll learn how to use the incredibly helpful enumerate()
function to find the indices of the highest value in a Python list.
Want to learn more about Python for-loops? Check out my in-depth tutorial that takes your from beginner to advanced for-loops user! Want to watch a video instead? Check out my YouTube tutorial here.
Find Index of Max Item in Python List with Enumerate
The Python enumerate() function is an incredible function that lets us iterate over an iterable item, such as a list, and access both the index and the item. This, of course, is very helpful since we’re trying to record the index positions of a given value in a list.
While we could use a for loop for this approach, we will use a list comprehension that allows us to be much more concise in our approach.
Let’s first see what the code looks like and then explore the why and how of it works:
# Get Indices of the Max Value from a List using enumerate()
a_list = [10, 11, 35, 14, 23, 9, 3, 35, 22]
indices = [index for index, item in enumerate(a_list) if item == max(a_list)]
print(indices)
# Returns: [2, 7]
In the code above, we:
- Loop over each index and item in our list
- If the item is equal to the maximum value of our list, we keep its index
- If the item isn’t equal to the highest value, then we do nothing
In the final section of this tutorial, you’ll learn how to use numpy
to find the indices of the max item of a list.
Want to learn more about Python list comprehensions? Check out this in-depth tutorial that covers off everything you need to know, with hands-on examples. More of a visual learner, check out my YouTube tutorial here.
Find Index of Max Item in Python List using numpy
In this final section, you’ll learn how to use numpy
in order to find the indices of the items with the highest values in a given Python list.
numpy
works well with one of its own data types, arrays
, which are list-like structures. One of the benefits of these arrays is that they come with many built-in functions and methods that normal lists do not.
One of these functions is the argmax()
function, which allows us to find the first instance of the largest value in the array.
# Get Index of the Max Value from a List using numpy
import numpy as np
a_list = [10, 11, 35, 14, 23, 9, 3, 35, 22]
an_array = np.array(a_list)
index = np.argmax(an_array)
print(index)
# Returns: 2
This works by:
- Converting the list to the array
- Using the
argmax()
function returns the index of the first maximum value
Want to learn more about Python f-strings? Check out my in-depth tutorial, which includes a step-by-step video to master Python f-strings!
Conclusion
In this tutorial, you learned how to find the index of the max value of a Python list. You learned how to do this if all values are unique or if duplicates can exist in different lists. You learned how to do this using the index()
function, as well as with a for loop. You also learned how to use the enumerate()
function in a Python list comprehension, and the popular data science library, numpy
.
To learn more about numpy
, check out the official documentation here.
Pingback: Python For Loop Tutorial - All You Need to Know! • datagy
def n_max(a, n):
”’ Returns the n largest items in list a. ”’
return [a.pop(a.index(max(a))) for _ in range(n)]
test = [7, 9, 10, 9, 8, 3, 4, 6, 2, 1]
print(n_max(test, 4))