In this tutorial, you’ll learn how to use Python to combine lists, including how to combine lists in many different ways. You’ll learn, for example, how to append two lists, combine lists sequentially, combine lists without duplicates, and more.
Being able to work with Python lists is an incredibly important skill. Python lists are mutable objects meaning that they can be changed. They can also contain duplicate values and be ordered in different ways. Because Python lists are such frequent objects, being able to manipulate them in different ways is a helpful skill to advance in your Python career.
The Quick Answer: Use the + Operator
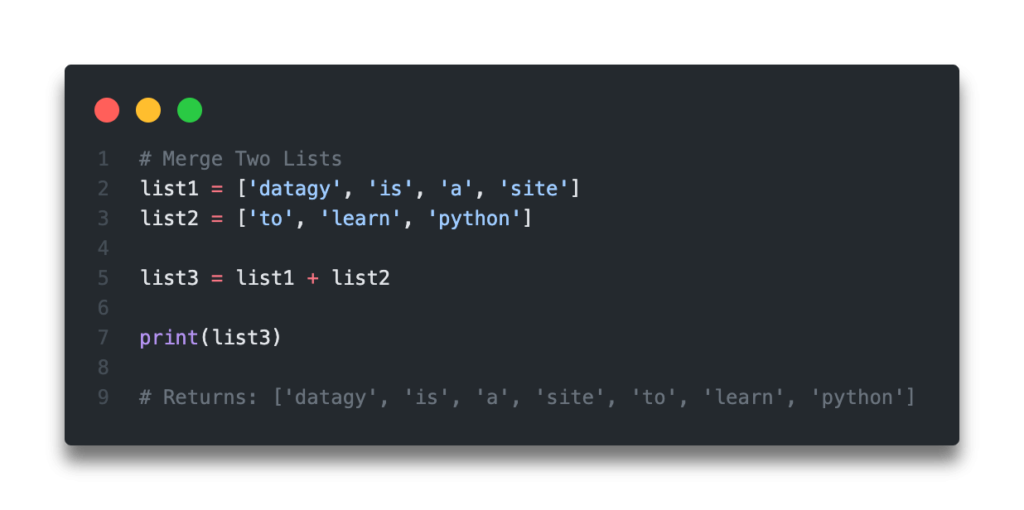
Table of Contents
Combine Python Lists
The easiest way to combine Python lists is to use either list unpacking or the simple +
operator.
Let’s take a look at using the +
operator first, since it’s syntactically much simpler and easier to understand. Let’s see how we can combine two lists:
# Merge Two Lists
list1 = ['datagy', 'is', 'a', 'site']
list2 = ['to', 'learn', 'python']
list3 = list1 + list2
print(list3)
# Returns: ['datagy', 'is', 'a', 'site', 'to', 'learn', 'python']
We can see here that when we print out third list that it combines the first and second.
Similarly, we can create a new list by unpacking all items from the lists we want to combine. By using the *
operator, we can access all the items in both lists and unpack them into a third.
Let’s see what this would look like:
# Merge Two Lists
list1 = ['datagy', 'is', 'a', 'site']
list2 = ['to', 'learn', 'python']
list3 = [*list1, *list2]
print(list3)
# Returns: ['datagy', 'is', 'a', 'site', 'to', 'learn', 'python']
In the next section, you’ll learn how to combine two lists in Python in an alternating fashion, using the Python zip()
function.
Want to learn more about Python f-strings? Check out my in-depth tutorial, which includes a step-by-step video to master Python f-strings!
Combine Python Lists Alternating Using Zip
When you combine Python lists, you may want to add items in an alternating method. For example, you may want to add the first item from both lists, then the second item, and so on.
In order to this, we can use the Python zip()
function. The zip function iterates over multiple items sequentially, allowing us to access the items in order.
Let’s see how we can use the zip()
function to combine Python lists in an alternating fashion:
# Merge Two Lists in Alternating Fashion
list1 = ['1a', '2a', '3a']
list2 = ['1b', '2b', '3b']
list3 = [item for sublist in zip(list1, list2) for item in sublist]
print(list3)
# Returns: ['1a', '1b', '2a', '2b', '3a', '3b']
The zip()
function creates a zip object, which is technically a generator object. When we turn this back into a list, we access all the items in the generator. Because of this, we can use the function to combine two Python lists in sequential order.
We then use a list comprehension to flatten the zip object. To learn more about how this works, check out my tutorial on flattening lists of lists.
In the next section, you’ll learn how to combine two Python lists without duplicates.
Want to learn how to use the Python zip()
function to iterate over two lists? This tutorial teaches you exactly what the zip()
function does and shows you some creative ways to use the function.
Combine Python Lists without Duplicates
Python lists allow us to have duplicate values in them – it’s one of the attributes that makes them special.
Another Python data structure, a set, is similar to a list in some ways, but cannot have duplicate items. We can use the set to remove any duplicates from a list, by converting a list into a set. To learn more about removing duplicates from a Python list, check out my tutorial here.
Let’s see how we can combine our lists and remove all duplicates by using a set:
# Combine Python Lists without Duplicates
list1 = [1, 2, 3, 4]
list2 = [4, 5, 6, 7]
list3 = list(set(list1 + list2))
print(list3)
# Returns: [1, 2, 3, 4, 5, 6, 7]
Let’s explore what we did here:
- We combined our lists using the
+
operator, - We then converted that list into a set to remove duplicates
- We then converted the set back into a list
In the next section, you’ll learn how to concatenate arrays using Python.
Need to automate renaming files? Check out this in-depth guide on using pathlib to rename files. More of a visual learner, the entire tutorial is also available as a video in the post!
Python Concatenate Arrays
numpy
, the popular Python data science library, has a list-like data structure called numpy arrays
. These arrays have a number of different methods to making working with them easier.
One of these methods is the np.concactenate()
method, which, well, concatenates two lists.
Let’s see how we can use Python and numpy
to do this:
# Concactenate Python Arrays
import numpy as np
list1 = [1,2,3]
list2 = [4,5,6]
array1 = np.array(list1)
array2 = np.array(list2)
array3 = np.concatenate((array1, array2.T))
print(array3)
# Returns: [1 2 3 4 5 6]
Let’s explore what we did here:
- We converted our lists into numpy arrays
- We then applied the concatenate method to the first array and passed in the second
In the next section, you’ll learn how to combine Python lists using a for loop.
Want to learn how to pretty print a JSON file using Python? Learn three different methods to accomplish this using this in-depth tutorial here.
Combine Python Lists with a For Loop
In this section, you’ll learn how to use a for loop to append items from one list to another. While this isn’t really approach that’s necessary in most cases, it does give you flexibility in terms of what items to include.
For example, using a for loop, you can skip certain items.
Let’s take a look at two examples: (1) where you combine all items, and (2) where you combine conditionally:
# Combine Two Python Lists with a For Loop
list1 = ['datagy', 'is', 'a', 'site']
list2 = ['to', 'learn', 'python']
# Combine all items
for item in list2:
list1.append(item)
print(list1)
# Returns: ['datagy', 'is', 'a', 'site', 'to', 'learn', 'python']
# Combine items conditionally
list1 = ['datagy', 'is', 'a', 'site']
list2 = ['to', 'learn', 'python']
for item in list2:
if len(item) > 3:
list1.append(item)
print(list1)
# Returns: ['datagy', 'is', 'a', 'site', 'learn', 'python']
Let’s explore what we did here in both examples:
- We looped over our second list appended each item to our first list
- In the conditional example, we checked for a condition. If the condition is met, then we append the item to the list. If it isn’t, then the item is skipped.
In the next section, you’ll l earn how to use a Python list comprehension to combine lists in Python.
Want to learn more about Python for-loops? Check out my in-depth tutorial that takes your from beginner to advanced for-loops user! Want to watch a video instead? Check out my YouTube tutorial here.
Combine Python Lists with a List Comprehension
We can also use a Python list comprehension to combine two lists in Python. This approach uses list comprehensions a little unconventionally: we really only use the list comprehension to loop over a list an append to another list.
Let’s see what this looks like:
# Combine Two Python Lists with a List Comprehension
list1 = ['datagy', 'is', 'a', 'site']
list2 = ['to', 'learn', 'python']
[list1.append(item) for item in list2]
print(list1)
# Returns: ['datagy', 'is', 'a', 'site', 'to', 'learn', 'python']
The reason that this approach is a bit strange is that the comprehension floats by itself, acting more as a statement than the traditional assignment of a new list. Syntactically, this approach is strange and doesn’t server much purpose that is different from a for loop.
In the next section, you’ll learn how to combine Python lists with only common elements.
Want to learn more about Python list comprehensions? Check out this in-depth tutorial that covers off everything you need to know, with hands-on examples. More of a visual learner, check out my YouTube tutorial here.
Combine Python Lists with Common Elements
There may the times when you want to only combine common elements between two Python lists, meaning the intersection between the two lists. I have an in-depth tutorial on this topic here, which goes into great details around how this approach works.
The approach shown below uses sets
to create unique elements across lists. The tutorial linked to above shows many different approaches to be able to accomplish this.
Let’s take a look at how to combine lists with only common elements:
# Combine common elements between two lists
list1 = [1, 2, 3, 4]
list2 = [3, 4, 5, 6]
list3 = list(set(list1).intersection(set(list2)))
print(list3)
# Returns: [3, 4]
Let’s explore what we’ve done here:
- Both lists are converted to sets
- The first set uses the
.intersection()
method and passes the second into its argument - The final set is returned as a list
In the next section, you’ll learn how to combine two lists using only unique elements across two lists.
Check out some other Python tutorials on datagy, including our complete guide to styling Pandas and our comprehensive overview of Pivot Tables in Pandas!
Combine Python Lists with Unique Elements Only
Similar to the example above, you may want to combine two lists and only keep unique elements across the two lists.
For this approach, we’ll again use sets, similar to the approach above, first checking for the intersection between two lists.
Let’s see how we can do this in Python and then dive into how it works:
# Combine unique elements between two lists
list1 = [1, 2, 3, 4]
list2 = [3, 4, 5, 6]
common_elements = list(set(list1).intersection(set(list2)))
combined = list1 + list2
for item in common_elements:
combined = [element for element in combined if element != item]
print(combined)
# Returns: [1, 2, 5, 6]
Let’s explore what we’ve done here:
- We found the intersection between the two lists and created a list called
common_elements
- We then combined our two lists into a list called
combined
- We then looped over the list of common elements
- For each item, we executed a list comprehension that looped over common element, excluding it from the combined list
The reason we use a list comprehension is that it allows us to check for duplicates. If we’d simply used a method, such as .remove()
, only the first instance would have been removed.
Want to learn how to use the Python zip()
function to iterate over two lists? This tutorial teaches you exactly what the zip()
function does and shows you some creative ways to use the function.
Conclusion
In this post, you learned how to use Python to combine two lists. You learned how to do this by simply combining two lists in order. You also learned how to combine lists in alternating sequence and without duplicates. You also learned how to use for loops and list comprehensions to combine Python lists. Finally, you learned how to combine lists with only common elements and with only unique elements.
To learn more about the Python list data structure, check out the official documentation here.