In this tutorial, you’ll learn how to calculate use Python to calculate the square root of a number, using the .sqrt()
function. You’ll learn how to do this with, and without, the popular math
library that comes built into Python. You’ll also learn what a square root is, what limitations there are of square roots, and how to calculate the integer square root using the math.isqrt()
function.
The Quick Answer: Use math.sqrt() to Calculate a Python Square Root
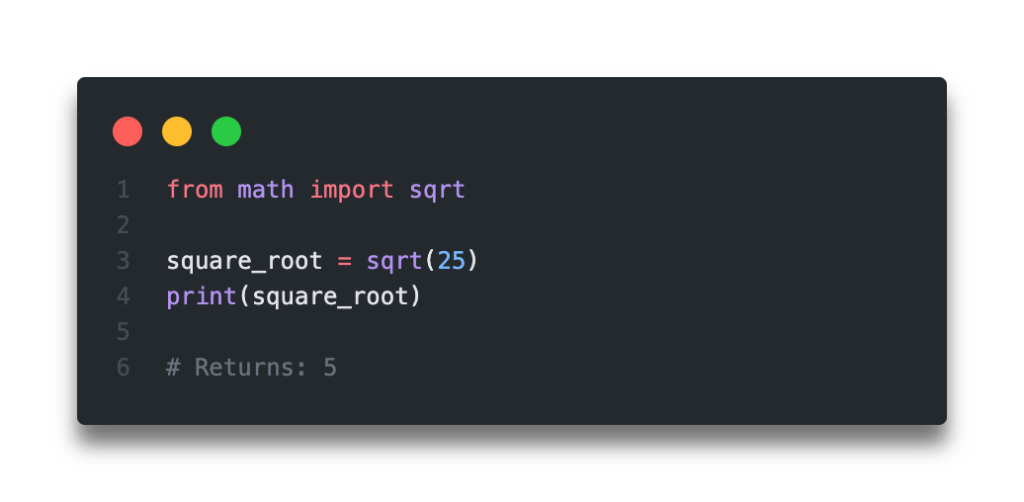
Let’s see what you’ll learn in this post!
Table of Contents
What is a Square Root?
In math, you often hear of the square of a number, which is often represented by a superscript 2. So, a square of a number n
, would be represented by n2
. The square of a number is calculated by multiplying the number by itself.
Because of this, the square of a number will always have some properties:
- It will always be a positive number (since the product of two negative numbers is a positive number)
- Can be either an integer or a floating point number
But why are we learning about squares, if this article is about the square root
? The square root is much easier to understand when you understand the square of a number. This is because the square root is, quite literally, the root of the square.
What I mean by this, is that the square root of n2
is n
. The square root of a number, say y
, is often represented as √y
. The square root is used in many different mathematical and scientific functions, such as the pythagorean theorem, which calculates the length of the hypotenuse of a right angle triangle.
Now that you have a solid understanding of what the square root is, let’s dive into how to calculate the square root using Python!
Want to learn something else? Want to learn how to calculate the standard deviation in Python? Check out my in-depth tutorial here!
Python Square Root Using math.sqrt
To calculate the square root in Python, you can use the built-in math
library’s sqrt()
function. This makes it very easy to write and to help readers of your code understand what it is you’re doing.
The sqrt()
function takes only a single parameter, which represents the value of which you want to calculate the square root. Let’s take a look at a practical example:
# Using the sqrt() function to calculate a square root
from math import sqrt
number = 25
square_root = sqrt(number)
print(square_root)
# Returns: 5.0
Let’s explore what we’ve done here:
- We imported
sqrt
frommath
- We declared a variable
number
, holding the integer value of 25 - We used the
sqrt
function to create a new variablesquare_root
- Finally, we printed the value of
square_root
, which gave us the value of 5.0
Something important to note here is that the square root returned is a floating point value. This is because for most numbers (other than those called perfect squares), the value returned is not a neat integer value. Later on in this tutorial, you’ll learn how to create an integer square root. If you want to skip ahead, click here.
Check out some other Python tutorials on datagy, including our complete guide to styling Pandas and our comprehensive overview of Pivot Tables in Pandas!
Python Square Root Using Exponentiation
In an earlier section of this tutorial, you learned that the square root is the base of a square number. Similarly, we can write the square root of a number n
as n1/2
.
In Python, we can raise any number to a particular power using the exponent operator **
.
Let’s see how we can get the Python square root without using the math
library:
# Use exponents to calculate a square root
number = 25
square_root = number**(1/2)
print(square_root)
# Returns: 5.0
You can see here that this returns the same value as if we had used the sqrt()
function.
Similarly, we could also have written number**0.5
.
Important tip: If you’re using Python 2, you will need to ensure you’re using float division and write number**(1./2)
. This is because Python 2 floors to an integer. As such, simply writing number**(1/2)
would actually result in number**(0)
.
Square Root Limitations – Negative Numbers and Zero
In the two sections below, you’ll learn about two special cases of square roots. In particular, you’ll learn how to calculate the square root of zero, as well as what happens when you try to calculate the square root of a negative number!
Calculate the Square Root of Zero Using Python
Now, let’s see how we can use Python to calculate the value of the square root of zero. We can do this, again, using the sqrt()
function:
# Calculating the square root of zero
from math import sqrt
number = 0
square_root = sqrt(number)
print(square_root)
# Returns: 0.0
We can see from the example above that calculating the square root of zero does not cause an error.
Calculating the Square Root of Negative Numbers with Python
Finally, let’s take a look at what happens when we try to calculate the square root of negative numbers with Python. You’ll remember, from the earlier section, that squares are always positive numbers. So, what happens when we try to take the square root of a negative number? Let’ give this a shot!
# Calculating the square root of a negative number
from math import sqrt
number = -25
square_root = sqrt(number)
print(square_root)
# Returns: ValueError: math domain error
We can see that trying to calculate the square root of a negative number returns a ValueError
. The reason that this happens is because the square root of negative numbers simply don’t exist!
In the next section, you’ll learn how to calculate the integer square root.
Python Integer Square Root
There may be times when you want to return an integer value when you are calculating the square root of a value. Keep in mind, that unless you’re working with specific numbers (“perfect squares”), this won’t be the true square root of that number.
Python’s math
library comes with a special function called isqrt()
, which allows you to calculate the integer square root of a number.
Let’s see how this is done:
# Calculating the integer square root with Python
from math import isqrt
number = 27
square_root = isqrt(number)
print(square_root)
# Returns: 5
Similar to our example of using the sqrt()
function, the isqrt()
returned a value. When we passed in 27, the function returned 5! This is odd, since that’s actually the square root of 25.
The reason for this is that the isqrt()
floors the value to its nearest integer.
Conclusion
In this post, you learned how to use Python to calculate a square root, both with and without the math.sqrt()
function. You also learned what a square root is, what some of its limitations are, as well as how to calculate a Python integer square root.
To learn more about the math.sqrt()
function, check out the official documentation here.
Pingback: Pandas: Convert Column Values to Strings • datagy
Pingback: Python: Return Multiple Values from a Function • datagy