In this post, you’ll learn how to find an index of a substring in a string, whether it’s the first substring or the last substring. You’ll also learn how to find every index of a substring in a string.
Knowing how to work with strings is an important skill in your Python journey. You’ll learn how to create a list of all the index positions where that substring occurs.
The Quick Answer:
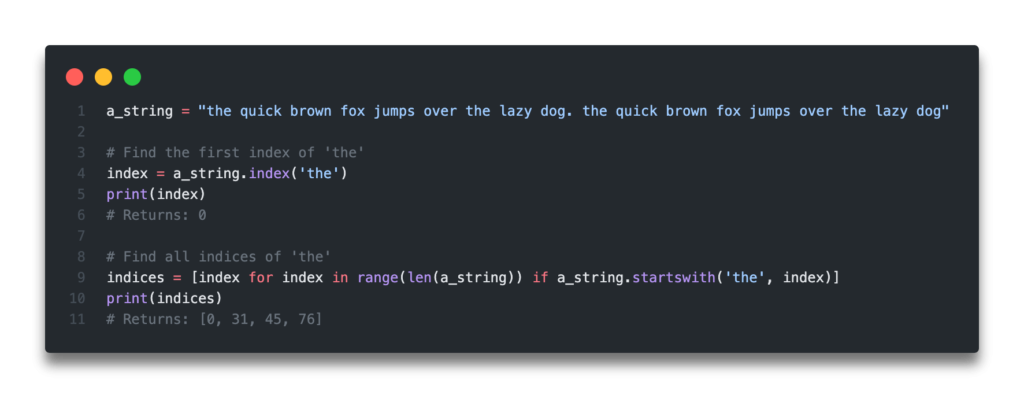
Table of Contents
How to Use Python to Find the First Index of a Substring in a String
If all you want to do is first index of a substring in a Python string, you can do this easily with the str.index()
method. This method comes built into Python, so there’s no need to import any packages.
Let’s take a look at how you can use Python to find the first index of a substring in a string:
a_string = "the quick brown fox jumps over the lazy dog. the quick brown fox jumps over the lazy dog"
# Find the first index of 'the'
index = a_string.index('the')
print(index)
# Returns: 0
We can see here that the .index()
method takes the parameter of the sub-string that we’re looking for. When we apply the method to our string a_string
, the result of that returns 0
. This means that the substring begins at index position 0, of our string (i.e., it’s the first word).
Let’s take a look at how you can find the last index of a substring in a Python string.
How to Use Python to Find the Last Index of a Substring in a String
There may be many times when you want to find the last index of a substring in a Python string. To accomplish this, we cannot use the .index()
string method. However, Python comes built in with a string method that searches right to left, meaning it’ll return the furthest right index. This is the .rindex()
method.
Let’s see how we can use the str.rindex()
method to find the last index of a substring in Python:
a_string = "the quick brown fox jumps over the lazy dog. the quick brown fox jumps over the lazy dog"
# Find the last index of 'the'
index = a_string.rindex('the')
print(index)
# Returns: 76
In the example above, we applied the .rindex()
method to the string to return the last index’s position of our substring.
How to Use Regular Expression (Regex) finditer to Find All Indices of a Substring in a Python String
The above examples both returned different indices, but both only returned a single index. There may be other times when you may want to return all indices of a substring in a Python string.
For this, we’ll use the popular regular expression library, re
. In particular, we’ll use the finditer
method, which helps you find an iteration.
Let’s see how we can use regular expressions to find all indices of a substring in a Python string:
import re
a_string = "the quick brown fox jumps over the lazy dog. the quick brown fox jumps over the lazy dog"
# Find all indices of 'the'
indices_object = re.finditer(pattern='the', string=a_string)
indices = [index.start() for index in indices_object]
print(indices)
# Returns: [0, 31, 45, 76]
This example has a few more moving parts. Let’s break down what we’ve done step by step:
- We imported
re
and set up our variablea_string
just as before - We then use
re.finditer
to create an iterable object containing all the matches - We then created a list comprehension to find the
.start()
value, meaning the starting index position of each match, within that - Finally, we printed our list of index start positions
In the next section, you’ll learn how to use a list comprehension in Python to find all indices of a substring in a string.
How to Use a Python List Comprehension to Find All Indices of a Substring in a String
Let’s take a look at how you can find all indices of a substring in a string in Python without using the regular expression library. We’ll accomplish this by using a list comprehension.
Want to learn more about Python list comprehensions? Check out my in-depth tutorial about Python list comprehensions here, which will teach you all you need to know!
Let’s see how we can accomplish this using a list comprehension:
a_string = "the quick brown fox jumps over the lazy dog. the quick brown fox jumps over the lazy dog"
# Find all indices of 'the'
indices = [index for index in range(len(a_string)) if a_string.startswith('the', index)]
print(indices)
# Returns: [0, 31, 45, 76]
Let’s take a look at how this list comprehension works:
- We iterate over the numbers from 0 through the length of the list
- We include the index position of that number if the substring that’s created by splitting our string from that index onwards, begins with our letter
- We get a list returned of all the instances where that substring occurs in our string
In the final section of this tutorial, you’ll learn how to build a custom function to return the indices of all substrings in our Python string.
Check out some other Python tutorials on datagy, including our complete guide to styling Pandas and our comprehensive overview of Pivot Tables in Pandas!
How to Build a Custom Function to Find All Indices of a Substring in a String in Python
Now that you’ve learned two different methods to return all indices of a substring in Python string, let’s learn how we can turn this into a custom Python function.
Why would we want to do this? Neither of the methods demonstrated above are really immediately clear a reader what they accomplish. This is where a function would come in handy, since it allows a future reader (who may, very well, be you!) know what your code is doing.
Let’s get started!
# Create a custom function to return the indices of all substrings in a Python string
a_string = "the quick brown fox jumps over the lazy dog. the quick brown fox jumps over the lazy dog"
def find_indices_of_substring(full_string, sub_string):
return [index for index in range(len(full_string)) if full_string.startswith(sub_string, index)]
indices = find_indices_of_substring(a_string, 'the')
print(indices)
# Returns: [0, 31, 45, 76]
In this sample custom function, we use used our list comprehension method of finding the indices of all substrings. The reason for this is that it does not create any additional dependencies.
Conclusion
In this post, you leaned how to use Python to find the first index, the last index, and all indices of a substring in a string. You learned how to do this with regular string methods, with regular expressions, list comprehensions, as well as a custom built function.
To learn more about the re.finditer()
method, check out the official documentation here.
Thanks for the information, it seems very useful. Although I have a question: in the for loop why is there the need to write ‘index’ before the ‘for’ word?
Thank you.
Great question! We’re using a list comprehension which works like a for loop. The syntax works like this:
[i for i in a_list]. We specify that we want to loop over each i in the a_list. The term we use doesn’t matter, but it’s the conventional syntax. You can learn more about them here: http://datagy.io/list-comprehensions-in-python/