In this tutorial, you’ll learn how to use Python to print an object’s attributes. Diving into the exciting world of object-oriented programming can seem an overwhelming task, when you’re just getting started with Python. Knowing how to access an object’s attributes, and be able to print all the attributes of a Python object, is an important skill to help you investigate your Python objects and, perhaps, even do a little bit of troubleshooting.
We’ll close the tutorial off by learning how to print out the attributes in a prettier format, using the pprint
module!
Let’s take a look at what you’ll learn!
The Quick Answer: Use the dir() Function
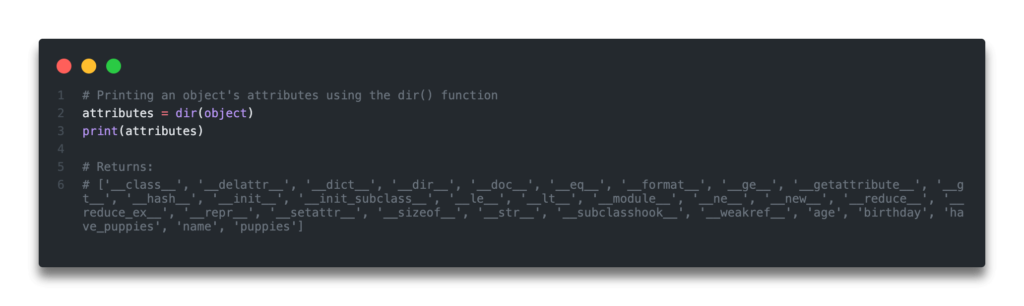
Table of Contents
What are Python Objects?
Python is an object-oriented language – because of this, much of everything in Python is an object. In order to create objects, we create classes, which are blueprints for objects. These classes define what attributes an object can have and what methods an object can have, i.e., what it can do.
Let’s create a fairly simple Python class that we can use throughout this tutorial. We’ll create a Dog
class, which will a few simple attributes and methods.
Let’s get started!
class Dog:
def __init__(self, name, age, puppies):
self.name = name
self.age = age
self.puppies = puppies
def birthday(self):
self.age += 1
def have_puppies(self, number_puppies):
self.have_puppies += number_puppies
What we’ve done here, is created our Dog
class, which has the instance attributes of name
, age
, and puppies
, and the methods of birthday()
and have_puppies()
.
Let’s now create an instance of this object:
teddy = Dog(name='Teddy', age=3, puppies=0)
We now have a Python object of the class Dog
, assigned to the variable teddy
. Let’s see how we can access some of its object attributes.
What are Python Object Attributes?
In this section, you’ll learn how to access a Python object’s attributes.
Based on the class definition above, we know that the object has some instance attributes – specifically, name, age, and puppies.
We can access an object’s instance attribute by suffixing the name of the attribute to the object.
Let’s print out teddy’s age:
print(teddy.name)
# Returns: Teddy
There may, however, be times when you want to see all the attributes available in an object. In the next two sections, you’ll learn how to find all the attributes of a Python object.
Use Python’s dir
to Print an Object’s Attributes
One of the easiest ways to access a Python object’s attributes is the dir()
function. This function is built-in directly into Python, so there’s no need to import any libraries.
Let’s take a look at how this function works:
# Printing an object's attributes using the dir() function
attributes = dir(teddy)
# Returns:
# ['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', 'age', 'birthday', 'have_puppies', 'name', 'puppies']
We can see here that this prints out of all the attributes of a Python object, including the ones that are defined in the class definition.
The dir()
function returns.a list of the names that exist in the current local scope returns the list of the names of the object’s valid attributes.
Let’s take a look at the vars()
function need to see a more in-depth way to print an object’s attributes.
Check out some other Python tutorials on datagy, including our complete guide to styling Pandas and our comprehensive overview of Pivot Tables in Pandas!
Use Python’s vars()
to Print an Object’s Attributes
The dir()
function, as shown above, prints all of the attributes of a Python object. Let’s say you only wanted to print the object’s instance attributes as well as their values, we can use the vars()
function.
Let’s see how this works:
print(vars(teddy))
# Same as print(teddy.__dict__)
# Returns:
# {'name': 'Teddy', 'age': 3, 'puppies': 0}
We can see from the above code that we’ve returned a dictionary of the instance attributes of our object teddy
.
The way that this works is actually by accessing the __dict__
attribute, which returns a dictionary of all the instance attributes.
We can also call this method on the class definition itself. Let’s see how that’s different from calling it on an object:
print(vars(Dog))
# Returns
# {'__module__': '__main__', '__init__': <function Dog.__init__ at 0x7ff59824ad30>, 'birthday': <function Dog.birthday at 0x7ff59824ae50>, 'have_puppies': <function Dog.have_puppies at 0x7ff59824aee0>, '__dict__': <attribute '__dict__' of 'Dog' objects>, '__weakref__': <attribute '__weakref__' of 'Dog' objects>, '__doc__': None}
We can see here that this actually returns significantly more than just calling the function on an object instance.
The dictionary also includes all the methods found within the class, as well as the other attributes provided by the dir()
method.
If we wanted to print this out prettier, we could use the pretty print pprint
module. Let’s see how we can do this:
import pprint
pprint.pprint(vars(Dog))
# Returns:
# {'__dict__': <attribute '__dict__' of 'Dog' objects>,
'__doc__': None,
'__init__': <function Dog.__init__ at 0x7fb4a0152d30>,
'__module__': '__main__',
'__weakref__': <attribute '__weakref__' of 'Dog' objects>,
'birthday': <function Dog.birthday at 0x7fb4a0152e50>,
'have_puppies': <function Dog.have_puppies at 0x7fb4a0152ee0>}
You’ve now learned how to print out all the attributes of a Python object in a more pretty format!
Conclusion
In this post, you learned how to print out the attributes of a Python object. You learned how to create a simple Python class and how to create an object. Then, you learned how to print out all the attributes of a Python object by using the dir()
and vars()
functions. Finally, you learned how to use the pprint
module in order to print out the attributes in a prettier format.
To learn more about the dir()
function, check out the official documentation here.