In this tutorial, you’ll learn how to use Python to get the length of a list (or, rather, its size). Knowing how to work with lists is an important skill for anyone using Python. Being able to get a Python list length, is particularly helpful. You’ll learn how to get the Python list length using both the built-in len()
function, a naive implementation for-loop method, how to check if a list is empty, and how to check the length of lists of lists.
The Quick Answer: Use len()
to get the Python list length
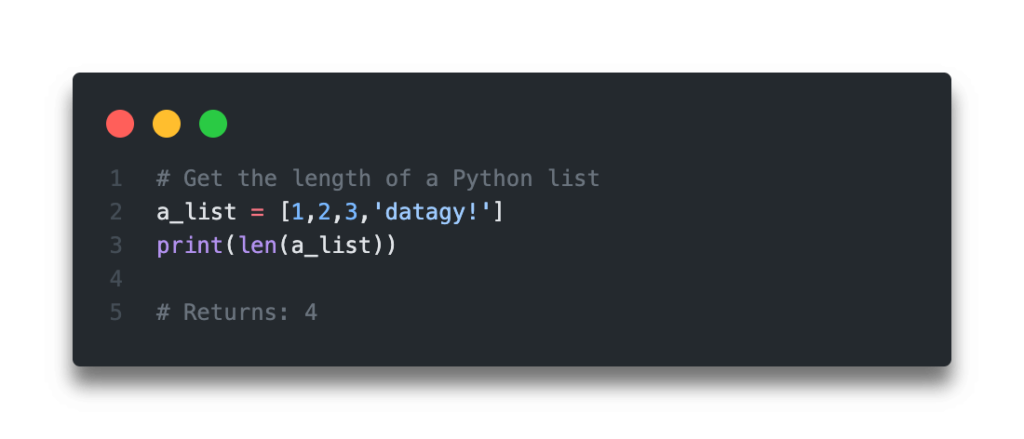
len()
function to get the length of a Python listTable of Contents
Python List Length using the len() function
The easiest (and most Pythonic) way to use Python to get the length or size of a list is to use the built-in len()
function. The function takes an iterable object as its only parameter and returns its length.
Let’s see how simple this can really be:
# Get the length of a Python list
a_list = [1,2,3,'datagy!']
print(len(a_list))
# Returns: 4
We can see here that by using the len()
function, we can easily return the length of a Python list.
In the next section you’ll learn how to use a for-loop naive implementation to get the size of a Python list.
Python List Length using a for-loop
While this approach is not recommended (definitely use the method above!), this for-loop method does allow us to understand an algorithm in terms of counting items in an iterable.
In order to do this, we iterate over each item in the list and add to a counter. Let’s see how we can accomplish this in Python:
# Get the length of a Python list
a_list = [1,2,3,'datagy!']
length = 0
for _ in a_list:
length += 1
print(length)
# Returns 4
This approach certainly isn’t as straightforward as using the built-in len()
function, but it does explain some algorithmic thinking around counting items in Python.
In the next section, you’ll learn how to easily check if a Python list is empty or not empty.
Want to learn more about Python for-loops? Check out my in-depth tutorial on Python for loops to learn all you need to know!
Check if a Python list is empty or not empty
In your programming journey, you’ll often encounter situations where you need to determine if a Python list is empty or not empty.
Now that you know how to get the length of a Python list, you can simply evaluate whether or not the length of a list is equal to zero or not:
# How to check if a Python list is empty
a_list = []
if len(a_list) != 0:
print("Not empty!")
else:
print("Empty!")
# Returns: Empty!
But Python makes it actually much easier to check whether a list is empty or not. Because the value of 0
actually evaluates to False
, and any other value evaluates to True
, we can simply write the following:
# An easier way to check if a list is empty
a_list = []
if a_list:
print("Not empty!")
else:
print("Empty!")
# Returns: Empty!
This is much cleaner in terms of writing and reading your code.
In the next section, you’ll learn how to get the length of Python lists of lists.
Learn to split a Python list into different-sized chunks, including how to turn them into sublists of their own, using this easy-to-follow tutorial.
Get Length of Python List of Lists
Working with Python lists of lists makes getting their length a little more complicated.
To better explain this, let’s take a look at an immediate example:
# Get the length of a list of lists
a_list_of_lists = [[1,2,3], [4,5,6], [7,8,9]]
print(len(a_list_of_lists))
# Returns: 3
We can see here, that the code (correctly) returns 3
. But what if we wanted to get the length of the all the items contained in the outer list?
In order to accomplish this, we can sum up the lengths of each individual list by way of using a Python list comprehension and the sum function.
Let’s see how this can be done:
# Get the length of a list of lists
a_list_of_lists = [[1,2,3], [4,5,6], [7,8,9]]
length = sum([len(sub_list) for sub_list in a_list_of_lists])
print(length)
# Returns: 9
Finally, let’s see how we can use Python to get the length of each list in a list of lists.
Want to learn more about Python list comprehensions? This in-depth tutorial will teach you all you need to know. More of a visual learner? A follow-along video is also included!
Get Length of Each List in a Python List of Lists
In the example above, you learned how to get the length of all the items in a Python list of lists. In this section, you’ll learn how to return a list that contains the lengths of each sublist in a list of lists.
We can do this, again, by way of a Python list comprehension. What we’ll do, is iterate over each sublist and determine its length. A keen eye will notice it’s the same method we applied above, simply without the sum()
function as a prefix.
Let’s take a look at how to do this:
# Get the length of each sublist in a list of lists
a_list_of_lists = [[1,2,3], [4,5,6], [7,8,9]]
lengths = [len(sublist) for sublist in a_list_of_lists]
print(lengths)
# Returns [3, 3, 3]
In this section, you learned how to use Python to get the length of each sublist in a list of lists.
Want to learn how to append items to a list? This tutorial will teach your four different ways to add items to your Python lists.
Conclusion
In this post, you learned how to use Python to calculate the length of a list. You also learned how to check if a Python list is empty or not. Finally, you learned how to calculate the length of a list of lists, as well as how to calculate the length of each list contained within a list of lists.
To learn more about the Python len()
function, check out the official documentation here.