In this tutorial, you’ll learn how to use Python to subtract two lists. You’ll learn how to do this using the popular data science library numpy
, for-loops, list comprehensions, and the helpful built-in zip()
function.
Being able to work with lists is an important skill for any Python developer, whether a beginner or an advanced Pythonista. Subtracting two lists is less intuitive than simply subtracting them. Because of this, check out the four different methods provided below to find easy ways to subtract two lists!
The Quick Answer: Use Numpy subtract()
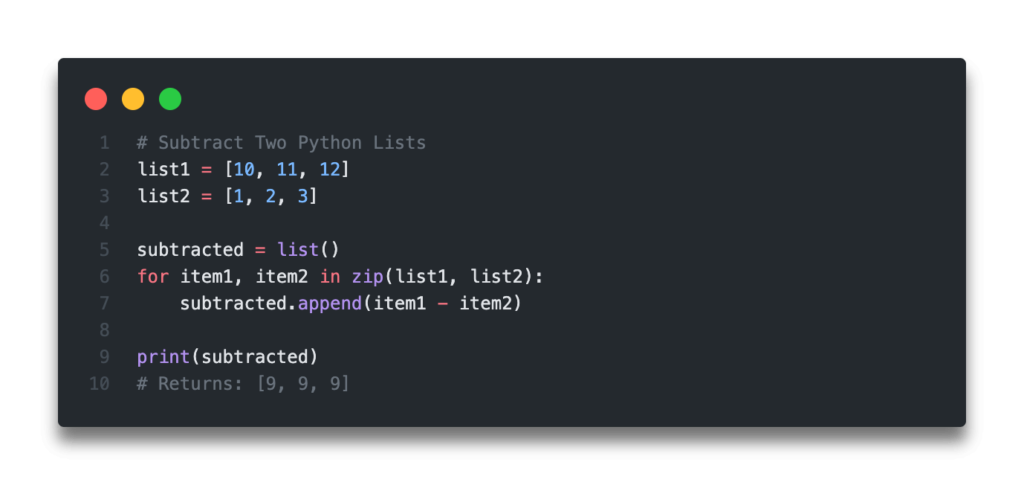
zip()
functionTable of Contents
Use Numpy to Subtract Two Python Lists
The popular numpy
library is often used for working in data science, and, as such, comes bundled with a ton of different helpful methods to manipulate numerical data. One of the primary advantages that numpy
provides is the array
object, which is very similar to the Python list object.
One of the methods that numpy
provides is the subtract()
method. The method takes two numpy array
s as input and provides element-wise subtractions between the two lists.
Let’s see how we can use numpy
and Python to subtract two lists:
# Subtract two lists with numpy
import numpy as np
list1 = [10, 11, 12]
list2 = [1, 2, 3]
array1 = np.array(list1)
array2 = np.array(list2)
subtracted_array = np.subtract(array1, array2)
subtracted = list(subtracted_array)
print(subtracted)
# Returns: [9, 9, 9]
Let’s take a look at what we’ve done here:
- We loaded our two lists,
list1
andlist2
and converted them into numpy arrays,array1
andarray2
- We then used the
np.subtract()
method to subtract the two lists - Finally, since we want to return a Python list, we used the
list()
method
In the next section, you’ll learn how to use the zip()
function to find the different between two lists element-wise.
Use Zip to Subtract Two Python Lists
The Python zip()
function is a built-in utility that makes working with different iterable objects incredibly easy. What the function does is iterate over different objects, element by element. Because of this, the zip()
function is the ideal candidate for finding the different between two lists element by element.
Let’s take a look at how we can use the Python zip()
function to subtract two lists:
# Subtract two lists with for loops
list1 = [10, 11, 12]
list2 = [1, 2, 3]
subtracted = list()
for item1, item2 in zip(list1, list2):
item = item1 - item2
subtracted.append(item)
print(subtracted)
# Returns: [9, 9, 9]
Let’s take a look at what we’ve done:
- We instantiated a new, empty list called
subtracted
that we’ll use to hold our subtracted values - We then iterate over two items returned during each iteration of the
zip()
output. - We assign the difference between these two items to
item
and append it to oursubtracted
list
Now, let’s take a look at how to use a for-loop to subtract two lists.
Want to learn how to use the Python zip()
function to iterate over two lists? This tutorial teaches you exactly what the zip()
function does and shows you some creative ways to use the function.
Python For Loops to Subtract Two Lists
Python for loops are incredibly helpful tools that let us repeat an action for a predetermined number of times. Because of this, we can loop over our two lists and find the different between their items.
Let’s see how we can use for loops to subtract lists:
# Subtract two lists with for loops
list1 = [10, 11, 12]
list2 = [1, 2, 3]
subtracted = list()
for i in range(len(list1)):
item = list1[i] - list2[i]
subtracted.append(item)
print(subtracted)
# Returns: [9, 9, 9]
Let’s take a look at what we’ve done with our for-loop method here:
- We created an empty list called
subtracted
- We then loop over the range from 0 through to the length of our first list
- We then assign the difference between each ith item of each list to our variable
item
- This
item
is then appended to our list
Finally, let’s
Want to learn more about Python for-loops? Check out my in-depth tutorial that takes your from beginner to advanced for-loops user! Want to watch a video instead? Check out my YouTube tutorial here.
Python List Comprehensions to Subtract Two Lists
Finally, let’s learn how we can use a list comprehension to subtract two lists. This method actually also uses the zip()
function to create a new list that subtracts the two lists.
Let’s take a look at how we can do this!
# Subtract two lists with zip()
list1 = [10, 11, 12]
list2 = [1, 2, 3]
subtracted = [element1 - element2 for (element1, element2) in zip(list1, list2)]
print(subtracted)
# Returns: [9, 9, 9]
Let’s take a look at what we’ve done here:
- We used a list comprehension to loop over the items in the zip() object of
list1
andlist2
- In this comprehension, we find the difference between the two elements
Want to learn more about Python list comprehensions? Check out this in-depth tutorial that covers off everything you need to know, with hands-on examples. More of a visual learner, check out my YouTube tutorial here.
Conclusion
In this post, you learned how to use to subtract two lists. You learned how to do this using the numpy.subract()
method, the zip()
function, a Python for-loop, and a Python list comprehension. Working with lists is an important skill that doesn’t always work as you’d expect, so being prepared for different challenges you might encounter is important.
To learn more about the numpy.subtract()
method, check out the official documentation.