In this tutorial, you’ll learn five different ways to use Python to check if a dictionary is empty. Learning to check if a dictionary is empty has many, many important uses, including in web development, where you may need to see if, say, a given user exists or an API call has been returned successfully.
You’ll learn a number of different ways to check if you Python dictionary is empty, as well as exploring the benefits and drawbacks of each approach.
The Quick Answer: Use if
to check if a Python dictionary is empty
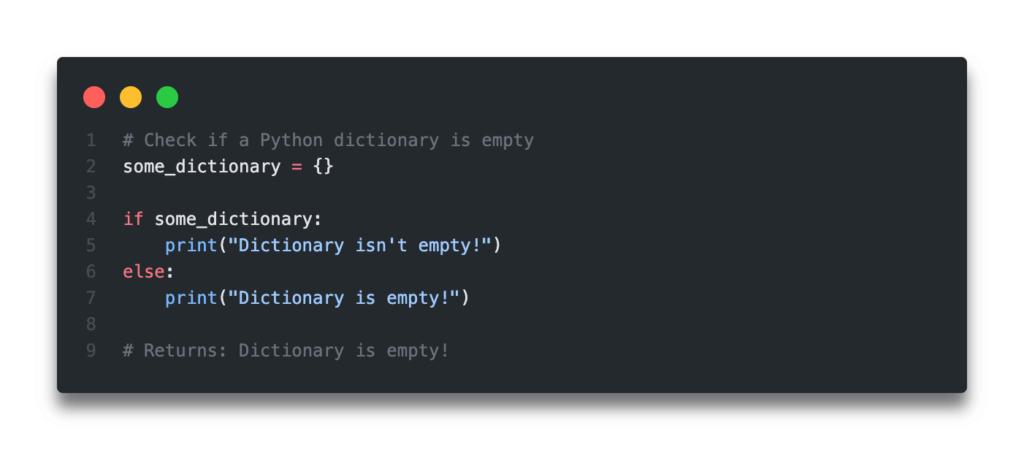
Table of Contents
What are Python Dictionaries?
Dictionaries in Python are one of the main, built-in data structures. They are collections of items, each consisting of a key:value
pairs. Python dictionaries are optimized to retrieve values, when the corresponding dictionary key is known.
Values themselves can be repeated, however keys must be unique. Values can also be of any data type (including other dictionaries), while keys must be immutable data types (such as strings, numbers, or tuples).
Let’s take a look at how we can create an empty dictionary in Python. We have two main options to create an empty dictionary in Python:
# Creating an empty Python dictionary
empty_dict1 = dict()
empty_dict2 = {}
Both of these methods create empty dictionaries. We can confirm this by printing out both of these dictionaries:
print(empty_dict1)
print(empty_dict2)
# Returns
# {}
# {}
We can see that both of these methods have returned empty dictionaries. Now, let’s dive into learning how we can use a Boolean function, bool()
, to see if a Python dictionary is empty.
Want to learn how to write Python dictionary comprehensions? Check out this in-depth tutorial in order to learn all you need to know as well as some examples for readers of all levels!
Check If a Python Dictionary is Empty Using a Boolean
A very simple way to check if a Python dictionary is empty, is to use the bool()
function, which evaluates whatever expression you place into it.
An interesting tidbit about Python dictionaries is that empty dictionaries will evaluate to False
, while dictionaries with at least one item will evaluate to True
. Because of this, we can use the bool()
function to see if a dictionary is empty.
Let’s give this is a shot:
# Using bool() to check if a Python dictionary is empty
not_empty_dict = {'some_key': 'datagy'}
empty_dict = {}
print("not_empty_dict is not empty: ", bool(not_empty_dict))
print("empty_dict is not empty: ", bool(empty_dict))
# Returns
# not_empty_dict is not empty: True
# empty_dict is not empty: False
We can see from the examples above, the using the bool()
function will return True
if a dictionary has any item(s) in it. However, the function will return False
, if the dictionary is empty.
In the next section, you’ll learn how you can trim this down even further.
Need to find the max value in a Python dictionary? Check out this tutorial which covers 4 different, easy-to-follow ways to find the max value in a Python dictionary.
Check if a Python Dictionary is Empty Using an If Statement
In the example above, we used the bool()
function to see if a Python dictionary is empty. In short, a dictionary passed into the bool()
function will return False
if a dictionary is empty.
Let’s see how we can see if a dictionary is empty using an if-statement
:
# Using an if statement to check if a Python dictionary is empty
empty_dict = {}
if empty_dict:
print("Dictionary is not empty!")
else:
print("Dictionary is empty!")
# Returns: Dictionary is empty!
The nice thing about Python if statements
is that they take any expression following if
and evaluate if it’s True
or False
. As we learned in the example above, an empty dictionary is technically False
, so we can even omit the bool()
function, if we’re passing into an if statement.
In the next section, you’ll learn how to check if a dictionary is empty by checking its length.
Want to learn how to do a VLOOKUP in Pandas? The trick is to use dictionaries! Learn how to do this in this in-depth tutorial with practical, follow-along examples.
Check if a Python Dictionary is Empty by Checking Its Length
Python dictionaries allow us to check their length, i.e., the number of keys the have, by using the built-in len()
function. Logically, a dictionary that is empty has, well, no items in it! Because of this, when we evaluate a dictionary’s length and it’s equal to zero, then we know the dictionary is empty!
Let’s see how we can do this:
# Checking if a dictionary is empty by checking its length
empty_dict = {}
if len(empty_dict) == 0:
print('This dictionary is empty!')
else:
print('This dictionary is not empty!')
# Returns: This dictionary is empty!
We can see here that by comparing a dictionary’s length to zero (0), we can use an if statement to determine whether a dictionary is empty and then split our program in different ways.
In this next section, you’ll learn how comparing a dictionary to an empty dictionary is an easy way to see if a dictionary is empty.
Want to remove a key from a dictionary? Learn 4 different ways to remove a key from a Python dictionary, including how to return the key’s value in this datagy tutorial!
Check if a Python Dictionary is Empty by Comparing it to An Empty Dictionary
In this section, you’ll learn another way to see if a dictionary is empty, by comparing your dictionary to an empty dictionary.
Let’s see what this looks like in Python:
# Checking if a dictionary is empty by comparing it to an empty dictionary
empty_dict = {}
if empty_dict == {}:
print('This dictionary is empty!')
else:
print('This dictionary is not empty!')
# Returns: This dictionary is empty!
This is a fairly straightforward example: we simply compare whether or not our dictionary is equal to an empty dictionary {}
. If this is the case, when we know it’s empty – if it’s not equal to that, well, then we know that it’s not empty.
In the next section, you’ll learn how to use the any()
function to see if a Python dict is empty.
Want to learn more? Check out this in-depth tutorial on learning 5 different ways to see if a key or a value exist in a Python dictionary!
Check if a Python Dictionary is Empty by Using the Any Function
For this final method, you’ll learn how to see if a Python dictionary is empty by using the any()
function.
The any()
function will check to see if any item of an iterable is True
. Since, as we learned earlier, an empty dictionary evaluates to False
, we can assume that the any()
function will return False
if a dictionary is empty.
Let’s see how we can accomplish checking if a dictionary is empty by using the Python any()
function:
# Checking if a dictionary is empty by using the any() function
empty_dict = {}
if any(empty_dict):
print('This dictionary is not empty!')
else:
print('This dictionary is empty!')
# Returns: This dictionary is empty!
Note that in the example above, we reversed the truthy-ness. This is because the first if condition will evaluate to False
, as nothing in the dictionary exists.
Want to create a Python dictionary from two lists? Check out this tutorial to learn how to do this useful skill.
Conclusion
In this post, you learned five different ways to check if a Python dictionary is empty. You first learned how to write a Python if
statement, or a boolean condition, to see if a dictionary is empty. You then learned to check a dictionary’s length, compared it to an empty dictionary, and finally used the any()
function to check if a dictionary is empty.
To learn more about Python dictionaries, check out the official documentation here.
Pingback: How Do You Write Null In A String In Python? – Almazrestaurant
The article was very good and informative .Thanks for the detailed explanation.
Thanks Sanjeev! I appreciate the feedback.