In this tutorial, you’ll learn how to use Python to transpose a list of lists, sometimes called a 2-dimensional array. By transposing a list of lists, we are essentially turning the rows of a matrix into its columns, and its columns into the rows. This is a very helpful skill to know when you’re learning machine learning.
In this tutorial, you’ll learn how to do this using for-loops, list comprehensions, the zip()
function, the itertools
library, as well as using the popular numpy
library to accomplish this task.
The Quick Answer: Use Numpy’s .T
Method
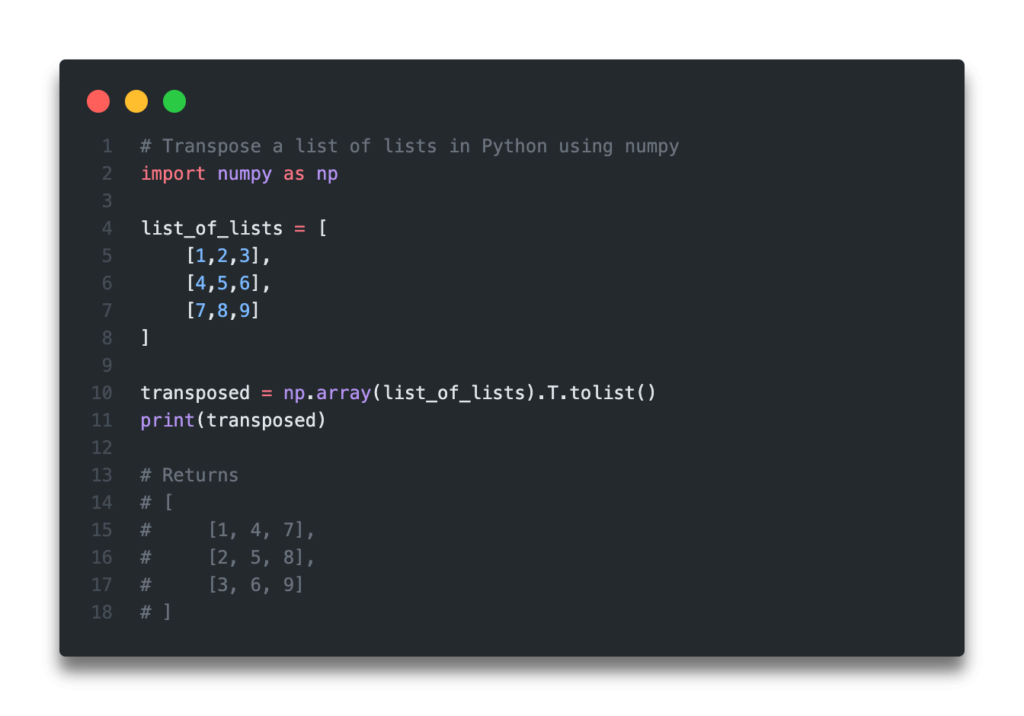
Table of Contents
What Does it Mean to Transpose a Python List of Lists?
Transposing arrays is a common function you need to do when you’re working on machine learning projects. But what exactly does it mean to transpose a list of lists in Python?
We can think of sublists in our list of lists in Python as the rows of a matrix. When we transpose a list of lists, we essentially turn the rows of our matrix into the columns, and turn the columns into the rows.
Let’s take a look at what this looks like visually:
original = [
[1,2,3],
[4,5,6],
[7,8,9]
]
transposed = [
[1, 4, 7],
[2, 5, 8],
[3, 6, 9]
]
Now let’s get started at learning how to transpose a two-dimensional array in Python.
Transpose a Python List of Lists using Numpy
Python comes with a great utility, numpy
, that makes working with numerical operations incredibly simple! Numpy comes packaged with different object types, one of which is the numpy array
. These arrays share many qualities with Python lists but also allow us to complete a number of helpful mathematical operations on them.
One of these operations is the transposing of data, using either the .transpose()
method, which can also be shortened to just .T
.
Before we can use any of these methods, however, we need to convert our list or our list of lists to a numpy array.
Let’s see how we can use numpy to transpose a list of lists:
# Transpose a list of lists in Python using numpy
import numpy as np
list_of_lists = [
[1,2,3],
[4,5,6],
[7,8,9]
]
array = np.array(list_of_lists)
transposed_array = array.T
transposed_list_of_lists = transposed_array.tolist()
# This is the same as: transposed = np.array(list_of_lists).T.tolist()
print(transposed_list_of_lists)
# Returns
# [
# [1, 4, 7],
# [2, 5, 8],
# [3, 6, 9]
# ]
From the example above, we can see how easy it is to use numpy to transpose a Python list of lists. What we did was:
- Convert our list of lists to a numpy array
- We then applied the
.T
method to transpose the data - Finally, we applied the
.tolist()
method to turn our array back to a list of lists
In the next section, you’ll learn how to transpose a two-dimensional array using the built-in zip()
function.
Want to learn how to transpose a Pandas Dataframe? Check out my in-depth tutorial with easy to follow along examples here.
Use the Zip Function to Transpose a List of Lists in Python
Let’s now take a look at how we can use the built-in zip()
function to transpose a list of lists in Python. The zip()
function is an incredibly helpful method that allows us to access the nth item of an iterable in sequence, as we loop over multiple iterable objects.
Now, this might sound a tad confusing, and it can be easier to see it in action than to understand it in theory, right off the bat. So let’s take a look at how we can do transpose a list of lists using the zip()
function:
# Transpose a list of lists in Python using numpy
import numpy as np
list_of_lists = [
[1,2,3],
[4,5,6],
[7,8,9]
]
transposed_tuples = list(zip(*list_of_lists))
# This looks like: [(1, 4, 7), (2, 5, 8), (3, 6, 9)]
transposed = [list(sublist) for sublist in transposed_tuples]
print(transposed)
# Returns
# [
# [1, 4, 7],
# [2, 5, 8],
# [3, 6, 9]
# ]
Let’s take a look at what we’ve done here:
- We created a list object using the
list()
function, out of zipping all the items in thelist_of_lists
object - Finally, we used a list comprehension to turn each item of the list into a list. By default, the zip objects will return tuples, which may not always be ideal.
In the next section, you’ll learn how to use the itertools
library to be able to turn lists of lists of different lengths into their transposed versions.
Want to dive a little deeper into the zip()
function in Python? Check out this in-depth tutorial that covers off how to zip two lists together in Python, with easy to follow examples!
Use Itertools to Transpose a Python List of Lists
Sometimes, when working with Python lists of lists, you’ll encounter sublists that are of different lengths. In these cases, the zip()
function will omit anything larger than the length of the shortest sublist.
Because of this, there are many times that we need to rely on the itertools
library in order to transpose lists of lists where items have different lengths. In particular, we’ll use the itertool’s zip_longest()
function to be able to include missing data in our transposed list of lists.
Again, this is much easier explained with an example, so let’s dive right in:
# Transpose a list of lists in Python using itertools
from itertools import zip_longest
list_of_lists = [
[1,2,3],
[4,5,6],
[7,8,9,10]
]
tranposed_tuples = zip_longest(*list_of_lists, fillvalue=None)
transposed_tuples_list = list(tranposed_tuples)
print(list(transposed_tuples_list))
# This returns: [(1, 4, 7), (2, 5, 8), (3, 6, 9), (None, None, 10)]
# To create a list of each item:
transposed = [list(sublist) for sublist in transposed_tuples_list]
print(transposed)
# Returns
# [
# [1, 4, 7],
# [2, 5, 8],
# [3, 6, 9],
# [None, None, 10]
# ]
We can see here, that a fourth row has been added. We used the fillvalue=
parameter to determine what to fill these missing gaps with. Much of this is the same as using the zip()
function, but it does allow us to work around the limitations of that function.
In the next two sections, you’ll learn two more methods, which are more naive implementations using for-loops and list comprehensions, in order to transpose lists of lists.
Transpose a Python List of Lists using a For Loop
Python for-loops as incredible tools to help you better understand how some algorithms may work. While they may not always be the most efficient way of getting something done, they can be easy to follow and document in terms of what your code is doing exactly.
Let’s see how we can implement a Python for loop to transpose a two-dimensional array of data:
# Transpose a list of lists in Python using for loops
list_of_lists = [
[1,2,3],
[4,5,6],
[7,8,9,10]
]
transposed = list()
for i in range(len(list_of_lists[0])):
row = list()
for sublist in list_of_lists:
row.append(sublist[i])
transposed.append(row)
print(transposed)
# Returns
# [
# [1, 4, 7],
# [2, 5, 8],
# [3, 6, 9]
# ]
What we’ve done here is the following:
- We initialize an empty list called
transposed
- We then loop over the length of the first list in the list of lists
- In this, we initialize a new empty list called
row
- We then loop over each ith item and append to our list
row
- Finally, we append that row to our
transposed
list - This then repeats for each item in the sublists
In the next section, you’ll learn how to turn this for-loop implementation into a Pythonic list comprehension.
Want to learn more about how to write for loops in Python? This tutorial will teach you all you need to know, including some advanced tips and tricks!
Use a List Comprehension to Transpose a List of Lists in Python
In many cases, a Python for loop can be turned a very abbreviated list comprehension. While we increase the brevity of our code, we may sacrifice the readability of our code.
Let’s take a look at how we can transpose a list of lists using list comprehensions:
# Transpose a list of lists in Python using a list comprehension
list_of_lists = [
[1,2,3],
[4,5,6],
[7,8,9,10]
]
transposed = [[row[i] for row in list_of_lists] for i in range(len(list_of_lists[0]))]
print(transposed)
# Returns
# [
# [1, 4, 7],
# [2, 5, 8],
# [3, 6, 9]
# ]
We can see here that we’ve saved quite a bit of space. We’ve not had to initialize two empty lists. That being said, this can be a bit more difficult to follow along with, especially as it’s much more difficult to provide guiding comments for each step of the way.
Want to learn more about Python list comprehensions? Check out my in-depth tutorial on them here. More of a visual learner? You’ll find an in-depth video on the topic inside the tutorial.
Conclusion
In this post, you learned how to transpose a Python list of lists, or a 2-dimensional array. You learned how to do this using the popular data science numpy
library, as well as using the built-in zip()
function, either alone or with the itertools
library. You also learned some more naive methods of accomplishing this, using both for loops as well as Python list comprehensions.
To learn more about the numpy
transpose method, check out the official documentation here.
That’s all well and great, but how to do this when you have over 100 million entries to transpose (in 5k+ files with the same data formats) and limited RAM? 🙂