In this post, you’ll learn how to use Python to get the last item from a list as well how as how to get the last n items from a list. You’ll learn a total of 4 different ways to accomplish this, learning how to find the best method for your unique solution. Let’s get started!
The Quick Answer: Use Negative Indexing
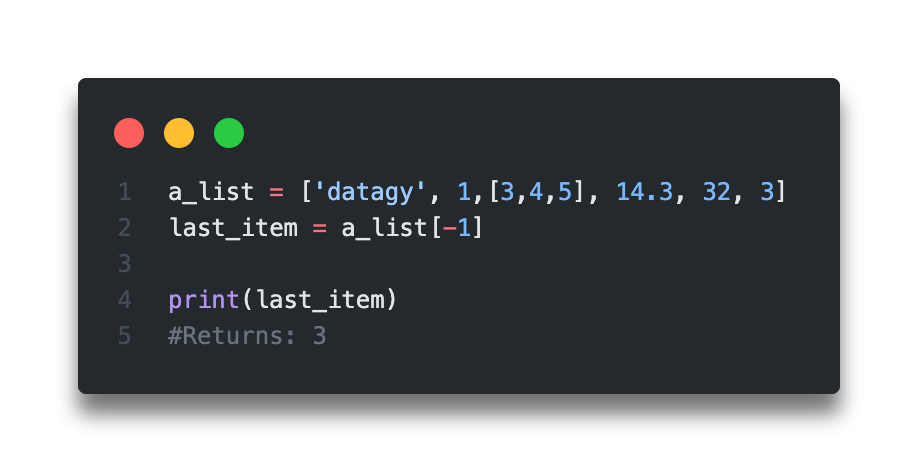
Table of Contents
How Does List Indexing Work in Python?
One of the unique attributes of Python lists is that they are ordered, meaning that their order matters. Because of this, we can access list items by their index positions.
Python lists begin at the 0th index, meaning that to get the first item, you would access index #0. Because of this, if you know how long a list is, you can access its last item by accessing that specific index. Say we have a list of length n, you would access the (n-1)th index.
This, of course, isn’t always practical because you don’t always know how long a list really is. Because of this, you can also access the list from the end, using negative indexing. By accessing the index of -1
, you would be accessing the last item in that list.
See the graphic below for an example of how indexes appear in a list:
Now that you have an understanding of how list indexing works in Python, let’s get started to access the last item in a list.
Python: Get the Last Item from a List Using Negative Indexing
Getting the last item in a Python list using negative indexing is very easy. We simply pull the item at the index of -1
to get the last item in a list.
Let’s see how this works in practice:
a_list = ['datagy', 1,[3,4,5], 14.3, 32, 3]
last_item = a_list[-1]
print(last_item)
#Returns: 3
Similarly, if you wanted to get the second last item, you could use the index of -2
, as shown below:
a_list = ['datagy', 1,[3,4,5], 14.3, 32, 3]
second_last_item = a_list[-2]
print(second_last_item)
#Returns: 32
Now let’s see how to get multiple items, specifically the last n items, from a list using negative indexing.
Get the Last n Items from a List Using Negative Indexing
In the section above, you learned how to use Python to get the last item from a list. Now, you’ll learn how to get the last n
items from a Python list.
Similar to the approach above, you’ll use negative indexing to access the last number of items.
One thing that’s great about Python is that if you want your indexing to go to the end of a list, you simply do not include a number at the end of the index.
Let’s say we wanted to return the last 2 items, we could write the following code:
a_list = ['datagy', 1,[3,4,5], 14.3, 32, 3]
items = a_list[-2:]
print(items)
#Returns: [32, 3]
Similar to the example above, we could have have written our index to be [-2:-1]
. However, omitting the -1
simply indicates that we want our index to go to the end of the list.
Interestingly, when slicing multiple items, even when they don’t exist, the whole list will be returned. For example, we shown below that slicing from the item -10
to the end doesn’t raise an error, but it also just omits the items that don’t exist.
a_list = ['datagy', 1,[3,4,5], 14.3, 32, 3]
items = a_list[-10:]
print(items)
#Returns: ['datagy', 1, [3, 4, 5], 14.3, 32, 3]
In the next section, you’ll learn how to get the last item form a Python list and remove it.
-
How to Perform T-Tests in Python (One- and Two-Sample)
In this post, you’ll learn how to perform t-tests in Python using the popular SciPy library. T-tests are used to test for statistical significance and can be hugely advantageous when working with smaller sample sizes. By the end of this tutorial, you’ll have learned the following: Understanding the T-Test The t-test, or often referred to…
How to Get the Last Item From a Python List and Remove It
Python has a built-in method, the .pop()
method, that let’s you use Python to get the last item from a list and remove it from that list. This can be helpful for many different items, such as game development and more.
Let’s take a look at how we can make this happen in practise:
a_list = ['datagy', 1,[3,4,5], 14.3, 32, 3]
last_item = a_list.pop()
print(f'{a_list=}')
print(f'{last_item=}')
# Returns:
# a_list=['datagy', 1, [3, 4, 5], 14.3, 32]
# last_item=3
You can see here that when we print out the list following applying the .pop()
method, that the last item has been removed. Furthermore, it has been assigned to the variable last_item
.
Tip! We’ve used some f-string formatting here to print out both the variable name and the variable contents simultaneously. Learn more about how to do this in my tutorial here (requires Python 3.8+).
In the next section, you’ll learn one more method to get the last item from a Python list using the reversed()
and next()
functions.
Use Reversed and Next to Get the Last Item From a List in Python
In this section, you’ll learn how to use the reversed()
and next()
functions to get the last item of a list.
The reversed()
function does exactly as it’s called – it returns an iterable in the reverse order of its original. The next()
function simply grabs the next iterable, which in this case is the first item. Because the list has been reversed, this results in the last item of the original list being returned.
Let’s see how this works in practise:
a_list = ['datagy', 1,[3,4,5], 14.3, 32, 3]
last_item = next(reversed(a_list))
print(last_item)
# Returns: 3
What you’ve done here is:
- Applied the
reverse()
function to reverse our list - Used the
next()
function to grab the first item - Finally, you assigned this to
last_item
and printed it out
In this section, you learned how to reverse a list and grab its first item to get the last item from a list.
Check out some other Python tutorials on datagy, including our complete guide to styling Pandas and our comprehensive overview of Pivot Tables in Pandas!
Conclusion
In this post, you learned how to use Python to get the last item from a list, as well as how to get the last n items from a list. You learned how to do this using negative list indexing, as well as how to do this using the reversed and next functions in Python.
To learn more about how lists are indexed in Python, check out the official documentation here.