In this tutorial, you’ll learn how to use Python to reverse a number. While this is similar to learning how to reverse a string in Python, which you can learn about here, reversing a number allows us to use math to reverse our number. You’ll learn how to use a Python while loop, string indexing, and how to create an easy-to-read function to reverse a number in Python.
The Quick Answer: Use a Python While Loop
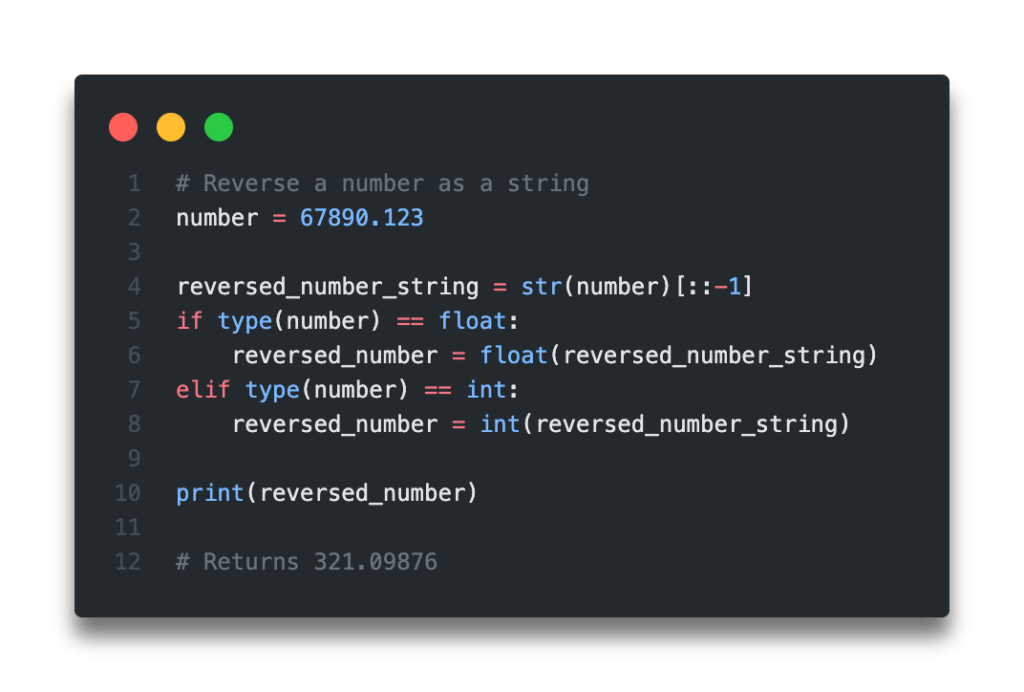
Table of Contents
Reverse a Python Number Using a While Loop
Python makes it easy to reverse a number by using a while loop. We can use a Python while loop with the help of both floor division and the modulus %
operator.
Let’s take a look at an example to see how this works and then dive into why this works:
# How to Reverse a Number with a While Loop
number = 67890
reversed_number = 0
while number != 0:
digit = number % 10
reversed_number = reversed_number * 10 + digit
number //= 10
print(reversed_number)
# Returns: 9876
Let’s break down what we do here:
- We instantiate two variables,
number
andreversed_number
. The first stores our original number and the second is given the value of0
- While our
number
variable is equal to anything by0
, we repeat our actions below - We instantiate
digit
, and assign it the modulus (remainder) of our number divided by 10 - We multiply our reversed number by 10 and add our digit
- Finally, we return the floored result of our number divided by 10 (this essentially removes the number on the right)
- This process is repeated until our original number is equal to zero
It’s important to note, this approach only works for integers and will not work for floats.
In the next section, you’ll learn how to use Python string indexing to reverse a number.
Reverse a Python Number Using String Indexing
Another way that we can reverse a number is to use string indexing. One of the benefits of this approach is that this approach will work with both integers and floats.
In order to make this work, we first turn the number into a string, reverse it, and turn it back into a number. Since we’ll need to convert it back to its original type, we need to first check the numbers type.
Let’s take a look at how we can accomplish this in Python:
# How to Reverse a Number with String Indexing
number = 67890.123
reversed_number_string = str(number)[::-1]
if type(number) == float:
reversed_number = float(reversed_number_string)
elif type(number) == int:
reversed_number = int(reversed_number_string)
print(reversed_number)
# Returns 321.09876
Python indexing allows us to iterate over an iterable object, such as a string. The third parameter is optional, but represents the step counter, meaning how to traverse an item. By default, the value is 1
, which means it goes from the first to the last. By using the value of -1
, we tell Python to generate a new string in its reverse.
We use type checking in order to determine what type of number to return back to.
In the next section, you’ll learn how to create a custom function that makes the code easier to follow and understand.
Reverse a Python Number Using a Custom Function
In this section, you’ll learn how to turn what you learned in the section above into an easy-to-read function. While the code may seem intuitive as we write it, our future readers may not agree. Because of this, we can turn our code into a function that makes it clear what our code is hoping to accomplish.
Let’s take a look at how we can accomplish this in Python:
def reverse_number(number):
"""Reverses a number (either float or int) and returns the appropriate type.
Args:
number (int|float): the number to reverse
Returns:
int|float: the reversed number
"""
if type(number) == float:
return float(str(number)[::-1])
elif type(number) == int:
return int(str(number)[::-1])
else:
print('Not an integer or float')
print(reverse_number(12345.43))
print(reverse_number(456))
# Returns:
# 34.54321
# 654
Our function accepts a single parameter, a number. The function first checks what the type is. If the type is either float
or int
, it reverses the number and returns it back to the same type. If the type is anything else, the function prints out that the type is neither a float nor an int.
Conclusion
In this post, you learned how to reverse a number using both math and string indexing. You also learned how to convert the string indexing method to a function that will make it clear to readers of your code what your code is doing.
If you want to learn more about string indexing in Python, check out the official documentation for strings here.
Additional Resources
To learn more about related topics, check out the resources below: