In this post, you’ll learn how to use Python to remove a character from a string. You’ll learn how to do this with the Python .replace()
method as well as the Python .translate()
method. Finally, you’ll learn how to limit how many characters get removed (say, if you only wanted to remove the first x number of instances of a character).
The Quick Answer: Use string.replace()
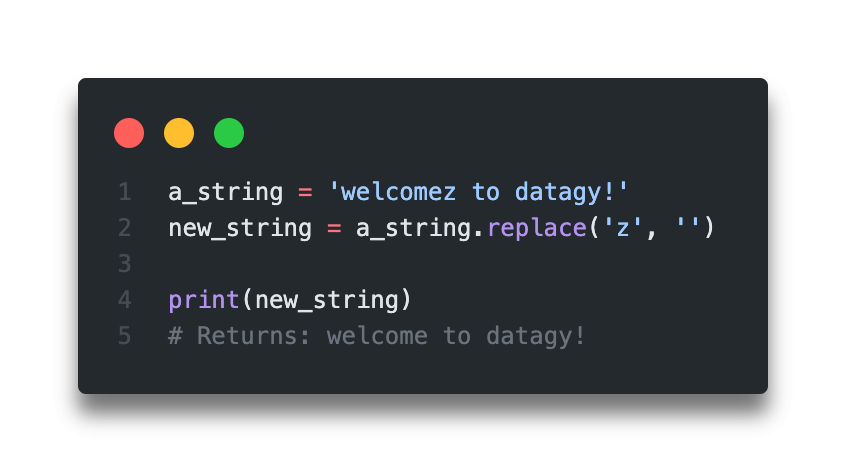
Table of Contents
Why Remove Characters from Strings in Python?
When you download data from different sources you’ll often receive very messy data. Much of your time will be spent cleaning that data and prepping it for analysis. Part of that data preparation will be cleaning your string and may involve removing certain characters.
Because of this being a very common challenge, this tutorial was developed to help you in your journey toward easier data analysis! Let’s get started!
Use the Replace Function to Remove Characters from a String in Python
Python comes built-in with a number of string methods. One of these methods is the .replace()
method that, well, lets you replace parts of your string. Let’s take a quick look at how the method is written:
str.replace(old, new, count)
When you append a .replace()
to a string, you identify the following parameters:
old=
: the string that you want to replace,new=
: the string you want to replace with, andcount=
: the number of replacements you want to make
Now that you’ve learned how the .replace()
method is written in Python, let’s take a look at an example. You’ll be given a string and will want to remove all of the ?
mark characters in the string:
old_string = 'h?ello, m?y? name? is ?nik!'
new_string = old_string.replace('?', '')
print(new_string)
# Returns: hello, my name is nik!
Let’s take a look at what we’ve done here to remove characters from a string in Python:
- We applied the .
replace()
method to our string,old_string
- We indicated we wanted to replace the
?
character with an empty string - We assigned this newly modified string to the variable
new_string
You can see here, just how easy it is to remove characters from a string in Python!
Now let’s learn how to use the Python string .translate()
method to do just this.
Use the Translate Function to Remove Characters from a String in Python
Similar to the example above, we can use the Python string .translate()
method to remove characters from a string.
This method is a bit more complicated and, generally, the .replace()
method is the preferred approach. The reason for this is that you need to define a translation table prior to actually being able to replace anything. Since this step can often be overkill and tedious for replacing only a single character. Because of this, we can use the ord() function to get a character’s Unicode value to simplify this process.
Let’s see how this can be done with the same example as above:
old_string = 'h?ello, m?y? name? is ?nik!'
new_string = old_string.translate({ord('?'):None})
print(new_string)
# Returns: hello, my name is nik!
Let’s explore what we’ve done here:
- We use the
ord()
function to return the Unicode value for whatever character we want to replace - We map this to a
None
value to make sure it removes it
You can see here that this is a bit more cumbersome than the previous method you learned.
Remove Only n Number of Characters from a String in Python
There may be some times that you want to only remove a certain number of characters from a string in Python. Thankfully, the Python .replace()
method allows us to easily do this using the count=
parameter. By passing in a non-zero number into this parameter we can specify how many characters we want to remove in Python.
This can be very helpful when you receive a string where you only need to remove the first iteration of a character, but others may be valid.
Let’s take a look at an example:
old_string = 'h?ello, my name is nik! how are you?'
new_string = old_string.replace('?', '', 1)
print(new_string)
# Returns: hello, my name is nik! how are you?
We can see here that by passing in count=1
, that only the very first replacement was made. Because of this, we were able to remove only one character in our Python string.
Remove Multiple Characters from a String in Python
There may also be times when you want to replace multiple different characters from a string in Python. While you could simply chain the method, this is unnecessarily repetitive and difficult to read.
Let’s take a look at how we can iterate over a string of different characters to remove those characters from a string in Python.
The reason we don’t need to loop over a list of strings is that strings themselves are iterable. We could pass in a list of characters, but we don’t need to. Let’s take a look at an example where we want to replace both the ?
and the !
characters from our original string:
a_string = 'h?ello, my name is nik! how are you?'
for character in '!?':
a_string = a_string.replace(character, '')
print(a_string)
# hello, my name is nik how are you
One thing you’ll notice here is that we are replacing the string with itself. If we didn’t do this (and, rather, replaced the string and assigned it to another variable), we’d end up only replacing a single character in the end.
We can also accomplish this using the regular expression library re
. We can pass in a group of characters to remove and replace them with a blank string.
Let’s take a look at how we can do this:
import re
old_string = 'h?ello, my name is nik! how are you?'
new_string = re.sub('[!?]', '', old_string)
print(new_string)
# Returns: hello, my name is nik how are you
What we’ve done here is pass in a string that contains a character class, meaning it’ll take any character contained within the square brackets []
.
The re
library makes it easy to pass in a number of different characters you want to replace and removes the need to run a for loop. This can be more efficient as the length of your string grows.
Conclusion
In this post, you learned how to remove characters from a string in Python using the string .replace()
method, the string .translate()
method, as well as using regular expression in re
.
To learn more about the regular expression .sub()
method, check out the official documentation here.
Additional Resources
To learn more about related topics, check out the resources below:
Hey, Nik!
There are two tiny mistakes in the penultimate section of code on this page:
http://datagy.io/python-remove-character-from-string/
The output of this code section (last line) should NOT contain characters of a question mark & of an exclamation point:
a_string = ‘h?ello, my name is nik! how are you?’
for character in ‘!?’:
a_string = a_string.replace(character, ”)
print(a_string)
# a_string: hello, my name is nik! how are you?
PS Btw thanks for your content: the articles are great, explanations are clear. Keep going!
Hi Dmitry,
Thanks so much for catching that! I’ve updated the article.
Thank you also for your feedback! It means a lot to me! 🙂
why
string.replace(“\n”,””)
and
string.translate({ord(‘\n’):None})
not working?
at least in pycharm
Thanks a lot for these contents.
I wish to know, if is there a method “remove” for string ??
Thanks so much for your comment! Because strings are immutable, there isn’t really a “remove” method for strings. Instead, you’re really replacing characters and creating a brand new string.