In this tutorial, you’ll learn how to use the Python floor()
function, which is used to round down with Python, to the nearest integer value. You’ll learn how this is different between Python 2 and Python 3, as well as some fringe cases when working with constants. You’ll also learn how the Python floor()
function is different than simply changing the type of a value to an int
using the int()
function. Finally, you’ll learn how floored division works in Python.
The Quick Answer: Python math.floor()
Rounds a Number Down
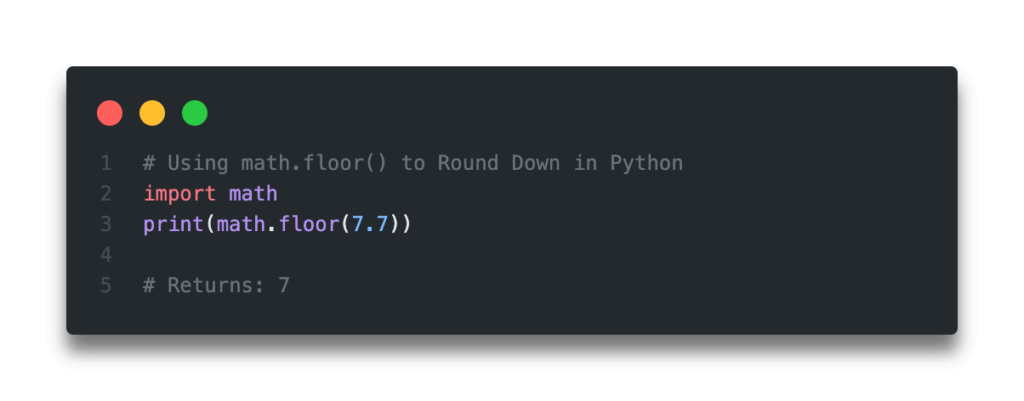
Table of Contents
What does it mean to round down?
Normally, when you round a number, the number is rounded to its closest integer. For example, 7.6 is closer to 8 than it is to 7. This is because it is only 0.4 away from 8, while it is 0.6 away from 7.
When you round a number down, however, the number is rounded to the closest integer than is not greater than the number. For example, 7.6 would round to 7.
Knowing how rounding a number down works has many useful applications, especially when building your own Python programs.
In the next section, you’ll learn how to use the math.floor()
function in Python to round a number down.
Want to learn how to round values down in a Pandas DataFrame? Check out this guide to rounding values in Pandas, including rounding values down.
Understanding Python floor()
Python has a built-in library, math
, which comes with a number of help mathematical tools like functions and constants. The function we’ll be using throughout this tutorial is the math.floor()
function, which floors a number, thereby returning the closest integer of a number, x, that is not greater than x.
Let’s take a look at how the function works:
# Understanding math.floor() in Python
import math
floored = math.floor(number)
In the example above, we create a new variable, floored
, by using the math.floor()
function, and passing in a variable number
.
Now that we have a good understanding of what the function looks like, let’s take a look at a few examples:
# Some examples of using Python's math.floor()
import math
number1 = 7.2
number2 = 7
number3 = -7.2
print(math.floor(number1))
print(math.floor(number2))
print(math.floor(number3))
# Returns:
# 7
# 7
# -8
Let’s now take a look at how this function behaves differently in Python 2 and Python 3.
Need to check if a key exists in a Python dictionary? Check out this tutorial, which teaches you five different ways of seeing if a key exists in a Python dictionary, including how to return a default value.
Difference Between Python Floor in Python 2 and Python 3
In Python 2, the math.floor()
function would return a rounded down floating point value (float
). Now, in Python 3, the math.floor()
function returns an integer
value.
If you wanted Python 3 to return a float
instead, you could simply write:
import math
rounded_down_float = float(math.floor(number))
In the next section, you’ll learn how the math.floor()
function works when applied to mathematical constants.
Want to learn how to use the Python zip()
function to iterate over two lists? This tutorial teaches you exactly what the zip()
function does and shows you some creative ways to use the function.
Using Python Floor with Constants
The Python math
library comes with a number of different constants. For example, the value of pi
can be found by using math.pi
.
Let’s see how the math.floor()
function interacts with some mathematical constants in Python:
import math
pi = math.pi
e = math.e
infinity = math.inf
print(math.floor(pi))
print(math.floor(e))
print(math.floor(infinity))
# Returns
# 3
# 2
# OverflowError: cannot convert float infinity to integer
We can see that while pi
and e
were able to be successfully used in the Python floor()
function, infinity
was not.
In the next section, you’ll learn the different between using int()
and floor()
in Python.
Want to learn more about Python for-loops? Check out my in-depth tutorial that takes your from beginner to advanced for-loops user! Want to watch a video instead? Check out my YouTube tutorial here.
Difference Between Int and Floor in Python
While reading this tutorial, it may seem that we are really only removing the pieces following a decimal. In this case, you may be wondering why we wouldn’t simply convert the float to an integer.
Let’s take a look at an example where this seems to work:
print(math.floor(3.7))
print(int(3.7))
# Returns:
# 3
# 3
In both cases, Python returned the same value.
But now let’s try this with a negative number:
import math
print(math.floor(-3.7))
print(int(-3.7))
# Returns:
# -4
# -3
When using negative numbers, Python will simply remove the values following the decimal place when using the int()
function. That, however, is not really rounding the value down, as a rounded down negative number jumps to the lower whole number.
Need to automate renaming files? Check out this in-depth guide on using pathlib to rename files. More of a visual learner, the entire tutorial is also available as a video in the post!
Python Floor Division
There are two types of division in Python, integer division and float division. I cover these two types of division of extension in this tutorial. One type of division, integer or floored division, uses the //
characters to divide values.
Similar to using the floor function in Python, this type of division returns the floored result of dividing the two values.
This is actually very similar to using float division and flooring the result.
Let’s take a look at what I mean with an example:
import math
a = 17
b = 5
floored_float_division = math.floor(a / b)
floored_division = a // b
print(floored_float_division)
print(floored_division)
# Returns
# 3
# 3
Because of this, we can actually say that math.floor(a / b) == a // b
. This can be a helpful item to know if you don’t want to import any additional packages for division.
Want to learn how to get a file’s extension in Python? This tutorial will teach you how to use the os and pathlib libraries to do just that!
Conclusion
In this post, you learned how to use the Python floor function to round down values in Python. You learned how this function behaves differently in Python 2 and Python 3. You also learned some limitations of the floor function when working with mathemical constants. Finally, you learned the differences between the int()
and floor()
functions, as well as how floored division works in Python.
To learn more about the math.floor()
function, check out the official documentation here.
Pingback: Python: Truncate a Float (6 Different Ways) • datagy
Pingback: Round Number to the Nearest Multiple in Python (2, 5, 10, etc.) • datagy