In this tutorial, you’ll learn how to use Python to convert a datetime to a string, using the Python strftime
function. You’ll also learn how to convert a datetime to a string using Python f-strings and string formatting.
Being able to to work with and manipulate dates is an important skill to learn as you advance on your Python journey. Many times, dates pose challenge for budding Pythonistas. As you download data from web APIs or need to manipulate local inputs, being able to return readable date formatted strings is an important skill to make your programs more user-friendly.
Want to learn how to convert strings to datetime objects in Python? Check out my in-depth tutorial that teaches you how to use the datetime.strptime() function.
The Quick Answer: Use Python datetime.strftime()
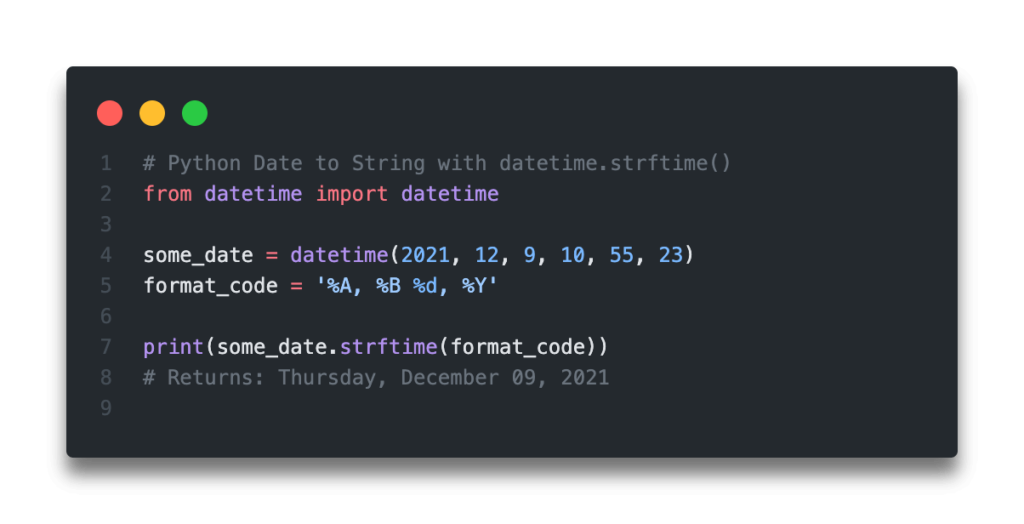
Table of Contents
Python Datetime to String using strftime
Python comes with a built-in library, datetime
, that is designed to work with dates and times. The library comes with a funtion, strftime()
specifically designed to convert datetime objects into strings.
At first glance, strftime()
looks like a bit of a mouthful to read. It stands for string from time.
The datetime.strftime()
method acts on a Python datetime object and accepts a single parameter, the format that you want your string to take.
Let’s take a look at what the Python strftime function looks like:
# Exploring the datetime.strftime() function
from datetime import datetime
some_date = datetime(2021, 12, 9, 10, 55, 23)
format_code = '%Y-%m-%d'
print(some_date.strftime(format_code))
# Returns: 2021-12-09
We can see here that we pass a specific format code into our parameter. The format codes follow the 1989 C Implementation, and may be familiar to users from other programming languages. See the section below for more format codes.
Python datetime objects contain a large amount of information, but there may be many times that you don’t want to return all of the information contain. Othertimes, you may want to extrapolate some additional information, such as the weekday name, from your string that may not be immediately visible from the datetime object.
Let’s take a look at this example.
# Getting the weekday from a particular date using datetime.strftime()
from datetime import datetime
some_date = datetime(2021, 12, 9, 10, 55, 23)
format_code = '%A'
print(some_date.strftime(format_code))
# Returns: Thursday
The function gives you control to even add additional string elements, such as commas or dashes, to allow you to properly format your strings.
Let’s take a look at an example that includes additional punctuation using the Python strftime method:
# Adding punctuation into your datetime to strings
from datetime import datetime
some_date = datetime(2021, 12, 9, 10, 55, 23)
format = '%A, %B %d, %Y'
print(some_date.strftime(format))
# Returns: Thursday, December 09, 2021
In the next section, you’ll learn some of the most important Python datetime format codes.
Python Datetime Format Codes
The datetime module uses a specific format codes in order to a date, time, or datetime object into a string. In the list below, you’ll learn a number of the most important format codes that you’ll encounter while using Python strftime:
%a
: Weekday in the locale’s abbreviated name (e.g., Mon)%A
: Weekday in the locale’s full name (e.g., Monday)%b
: month as locale’s abbreviated name (e.g., Dec)%B
: month as locale’s full name (e.g., December)%m
: month as zero-padded decimal number (e.g., 01)%d
: day as a zero-padded decimal number (e.g., 09)%y
: year without century (e.g., 2021)%Y
: year with century (e.g., 21)
For example, if want to create a string that looks like this: ‘Monday June 3, 2021’, you’d write ‘%A %B %d, %Y’ for your format code.
As shown in the section above, you’re able to include additional punctuation and seperators in order to make your strings more readable.
To see the rest of the format codes, check out the official documentation here.
Convert Python Timestamp to String
Using timestamps to store datetime information is a very common practice, especially in database design. The Python timestamp is represented by the Unix timestamp, which is the number of seconds between a given datetime and January 1, 1970 in UTC.
You need to first convert the timestamp to a datetime, but, we can accomplish this inline, so that we don’t need to generate a new variable first.
Let’s see how this works in Python:
# Convert a Python timestamp to a string
from datetime import datetime
timestamp = 1639062683
print(datetime.fromtimestamp(timestamp).strftime('%A %B %d, %Y'))
# Returns: Thursday December 09, 2021
In the next section, you’ll learn how to use locale specific information to create string from Python datetime objects.
Using Locales to Create Python Datetime Strings
Python will use a system’s locales to determine how to output a string. For example, if your system is set to English it will write Monday, while if your system is set to French, it will write Lundi.
Furthermore, Python takes this one step further. There are three special format codes, %c
, %x
and %X
, which will output strings based on a system’s locale.
Let’s see what these three look like on an English US system, when using Python strftime()
to convert dates to strings.
# Using system locale's in Python strftime
from datetime import datetime
some_date = datetime(2021, 12, 9, 12, 12, 23)
print(some_date.strftime('%c'))
print(some_date.strftime('%x'))
print(some_date.strftime('%X'))
# Returns:
# Thu Dec 9 12:12:23 2021
# 12/09/21
# 12:12:23
Using these shortcodes can save you quite a bit of time, especially when wanting to output in a regionally-appropriate setting. Otherwise, you may first need to identify a system’s locale and write complex if statements.
In the next section, you’ll learn how to use Python f-strings to convert a datetime to a string.
Using Python f-strings to Convert Datetime to Strings
Python f-strings were introduced in Python 3.6 and are technically called formatted string literals
. One of the unique attributes of Python f-strings is that they allow us to use format codes to output data in different ways.
In order to use Python f-strings to convert a datetime to a string, we can simply use the dt
format code. Following this format code, we can then use the datetime format codes to output our string as we choose.
Let’s take a look at what this looks like by using an example:
# Using Python f-strings to convert a date to a string
from datetime import datetime
some_date = datetime(2021, 12, 9, 12, 12, 23)
fstring = f'{some_date:%Y-%m-%d}'
print(fstring)
# Returns: 2021-12-09
In the next section, you’ll learn how to use Python string formatting to convert a Python date to a string.
Using Python String Formatting to Convert Datetime to Strings
If you’re using an older version of Python that doesn’t support Python f-strings, you can also use traditional string formatting to convert a date to a string in Python. This type of formatting is supported in Python versions 2.6 and higher.
Similar to the example above, the same datetime format codes are supported.
Let’s take a look at how we can use string formatting to convert a Python date to a time:
# Using string formatting to convert a date to a string in Python
from datetime import datetime
some_date = datetime(2021, 12, 9, 12, 12, 23)
string_formatting = '{:%b %d, %Y}'.format(some_date)
print(string_formatting)
# Returns: Dec 09, 2021
Note here that you need to use the colon :
character prior to including the format, to ensure that your format codes are accepted.
Conclusion
In this post, you learned how to use Python to convert a date, time, and datetime object to a string. You learned how to use the Python strftime()
method to convert a date to a string. You also learned how to use Python f-strings and Python string formatting to convert a date to a string.
To learn more about the .strftime()
method, check out the official documentation here.
Additional Resources
To learn more about related topics, check out the tutorials below:
Pingback: Pandas Datetime to Date Parts (Month, Year, etc.) • datagy