In this tutorial, you’ll learn how to use Python to concatenate a string and an int (integer). Normally, string concatenation is done in Python using the +
operator. However, when working with integers, the +
represents addition. Because of this, Python will raise an error, a TypeError
to be exact, when the program is run.
By the end of reading this tutorial, you’ll have learned how to use Python to concatenate a string and an int using a number of methods. You’ll have learned how to use the str()
function, the .format()
method, %
format specifier, and – my personal favourite – Python f-strings.
The Quick Answer: Use f-strings or str() to Concatenate Strings and Ints in Python
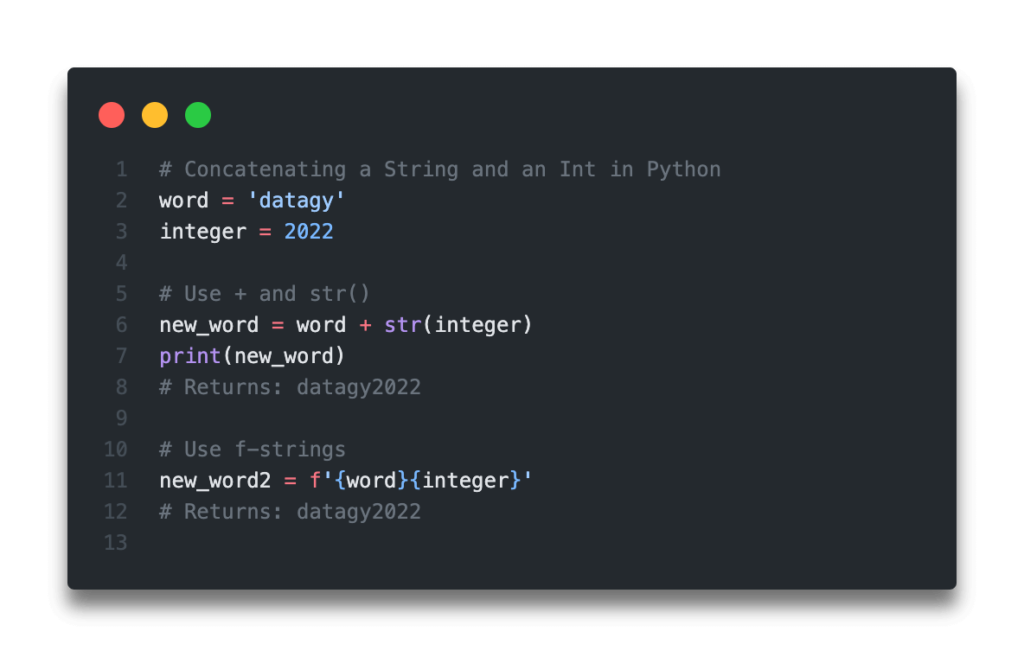
Table of Contents
Concatenate a String and an Int in Python with +
In many programming languages, concatenating a string and an integer using the +
operator will work seamlessly. The language will handle the conversion of the integer into a string and the program will run fine. In Python, however, this is not the case. Due to the dynamic typing nature of Python, the language cannot determine if we want to convert the string to an integer and add the values, or concatenate a string and an int. Because of this, the program will encounter a TypeError
.
Let’s see what this looks like when we simply try to concatenate a string and an integer in Python using the +
operator:
# Trying to Concatenate a String and an Int in Python
word = 'datagy'
integer = 2022
new_word = word + integer
# Returns: TypeError: can only concatenate str (not "int") to str
The TypeError
that’s raised specifically tells us that a string and an integer cannot be concatenated. Because of this, we first need to convert our integer into a string. We can do this using the string()
function, which will take an input and convert it into a string, if possible.
Let’s try running our running program again, but this time converting the int into a string first:
# Concatenating a String and an Int in Python with +
word = 'datagy'
integer = 2022
new_word = word + str(integer)
print(new_word)
# Returns: datagy2022
We can see that by first converting the integer into a string using the string()
function, that we were able to successfully concatenate the string and integer.
In the next section, you’ll learn how to use Python f-strings to combine a string and an integer.
Concatenate a String and an Int in Python with f-strings
Python f-strings were introduced in version 3.6 of Python and introduced a much more modern way to interpolate strings and expressions in strings. F-strings in Python are created by prepending the letter f
or F
in front of the opening quotes of a string. Doing so allows you to include expressions or variables inside of curly braces that are evaluated and converted to strings at runtime.
Because of the nature of f-strings evaluating expressions into strings, we can use them to easily concatenate strings and integers. Let’s take a look at what this looks like:
# Concatenating a String and an Int in Python with f-strings
word = 'datagy'
integer = 2022
new_word = f'{word}{integer}'
print(new_word)
# Returns: datagy2022
One of the great things about f-strings in Python is how much more readable they are. They provide us with a way to make it immediately clear which variables are being joined and how they’re being joined. We don’t need to wonder about why we’re converting an integer into a string, but just know that it’ll happen.
In the next section, you’ll learn how to use the .format()
method to combine a string and an integer in Python.
Concatenate a String and an Int in Python with format
The Python .format()
method works similar to f-strings in that it uses curly braces to insert variables into strings. It’s available in versions as far back as Python 2.7, so if you’re working with older version this is an approach you can use.
Similar to Python f-strings, we don’t need to worry about first converting our integer into a string to concatenate it. We can simply pass in the value or the variable that’s holding the integer.
Let’s see what this looks like:
# Concatenating a String and an Int in Python with .format
word = 'datagy'
integer = 2022
new_word = '{}{}'.format(word, integer)
print(new_word)
# Returns: datagy2022
We can see here that this approach returns the desired result. While this approach works just as well as the others, the string .format()
method can be a little difficult to read. This is because the values placed into the placeholders are not immediately visible.
In the next section, you’ll learn how to concatenate a string an int in Python using the %
operator.
Concatenate a String and an Int in Python with %
In this final section, you’ll learn how to use %
operator to concatenate a string and an int in Python. The %
operator represents an older style of string interpolation in Python. We place a %s
into our strings as placeholders for different values, similar to including the curly braces in the example above.
Let’s see how we can combine a string and an integer in Python:
# Concatenating a String and an Int in Python with %
word = 'datagy'
integer = 2022
new_word = '%s%s' % (word, integer)
print(new_word)
# Returns: datagy2022
We can see that this returns the same, expected result. This approach, however, is the least readable of the four approaches covered here. It’s included here more for completeness, rather than as a suggested approach.
Conclusion
In this tutorial, you learned how to use Python to concatenate a string and an int. You learned why this is not as intuitive as in other languages, as well as four different ways to accomplish this. You learned how to use the +
operator with the string()
function, how to use Python f-strings, and how to use the .format()
method and the %
operator for string interpolation.
To learn more about the Python string()
function, check out the official documentation here.
Related Tutorials
Check out some of these related tutorials: