In this tutorial, you’ll learn how to resize an image in Python using the Pillow library. You’ll learn how to resize individual images and multiple images in bulk. You’ll also learn how to specify a percentage to scale by, a maximum width, and a maximum height.
By the end of this tutorial, you’ll have learned:
- How to use the PIL library to open and resize an image
- How to use Python to resize images in bulk
- How to maintain an aspect ratio while resizing images in Python
Table of Contents
How to open an Image with Pillow
In order to resize an image using Python, we first need to open the image that we’re working with. For this, we can use the Pillow library. The library isn’t part of the standard Python library. Because of this, we first need to install it. This can be done using either pip
or conda
, as shown below:
# Installing the Pillow library
pip install pillow
# conda install pillow
Now that we have the library installed, we can load an image using Pillow. This can be done using the Image
module in Pillow:
# Opening an Image using Pillow
from PIL import Image
with Image.open ('/Users/datagy/Desktop/Original.png') as im:
im.show()
Running this code using a context manager safely manages memory. Once the indented code is done running, the image is closed and the memory is safely purged.
Running the code shows the following image:
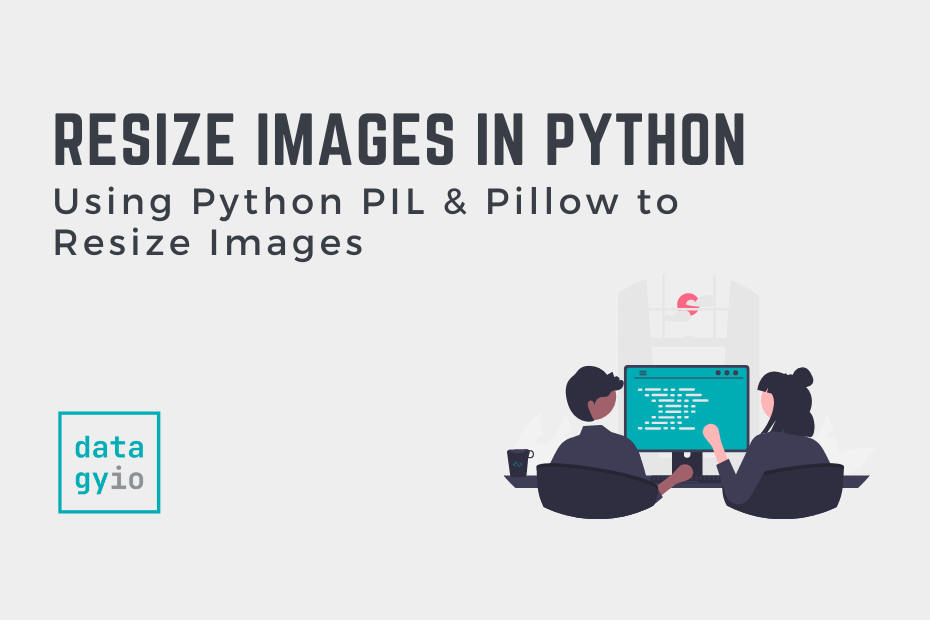
We can check the current dimensions of the image by using the .size
attribute. The attribute returns a tuple containing the width and height of the image:
# Getting Dimensions of an Image in Pillow
from PIL import Image
with Image.open ('/Users/datagy/Desktop/Original.png') as im:
print(im.size)
# Returns: (930, 620)
Now that we have safely opened the image, we can start on resizing the image using Pillow.
How to Resize an Image with Pillow resize
The Python Pillow library provides two ways in which to resize an image: resize()
and thumbnail()
. In this section, we’ll use the Pillow resize method to resize an image. This method allows you to:
- Upscale and downscale an image, by providing specific dimensions
- Resize an image even the aspect ratio doesn’t match the original image
Let’s take a look at the Pillow .resize()
method:
# The Python Pillow .resize() Method
Image.resize(
size, # Tuple representing size
resample=None, # Optional resampling filter
box=None, # Optional bounding box to resize
reducing_gap=None # Optional optimization
)
To keep things simple, we’ll focus on the size=
parameter. This allows us to pass in the new dimensions in a tuple of length two.
Let’s see how we can pass in a tuple containing the new width and height of the image:
# Resizing an Image using Pillow .resize()
from PIL import Image
with Image.open ('/Users/datagy/Desktop/Original.png') as im:
resized = im.resize((600,400))
resized.show()
Running this code changes the dimensions of the image to (600,400)
. This returns the following image:
Using the .show()
method allows us to open the image. If we wanted to save the image, we could instead write:
# Saving a Resized Image in Python Pillow
from PIL import Image
with Image.open ('/Users/datagy/Desktop/Original.png') as im:
resized = im.resize((600,400))
resized.save('/Users/datagy/Desktop/Resized.png')
Resizing an Image with Pillow to a Certain Percentage
There may be times when you simply want to resize an image to be a percentage of the original size. This can be done by using a custom function to calculate the tuple size that you want to use.
Let’s see how we can use a custom function to define the percentage that you want to resize an image by:
# Resizing an Image by Percentage
from PIL import Image
def resize_by_percentage(image, outfile, percentage):
with Image.open (image) as im:
width, height = im.size
resized_dimensions = (int(width * percentage), int(height * percentage))
resized = im.resize(resized_dimensions)
resized.save(outfile)
resize_by_percentage('/Users/datagy/Desktop/Original.png', '/Users/datagy/Desktop/Resized.png', 0.5)
Let’s break down what we did here:
- We defined a new function that takes three parameters: the original file path, the new file path, and the percentage by which to resize
- The image is opened and the dimensions are defined
- The dimensions are resized by the percentage that’s defined
- The
.resize()
method is used to resize the image - Finally, the image is saved using the
.save()
method
Running the above function generates the following image:
How to Resize an Image with Pillow thumbnail
The Pillow thumbnail method returns an image that is scaled to its original aspect ratio. The method allows you to pass in a tuple of values that represent the maximum width and height of the image that is meant to be generated.
This allows you to ensure that images have either a consistent width or height when working with multiple images. Let’s see how we can use the .thumbail()
method to resize our image.
The tuple that you pass in represents the maximum size of an image, on either dimension. So passing in (300, 200)
would generate an image that is no bigger than 300px wide or 200px wide.
Let’s apply this method to resize our image and find the resulting dimensions:
# Using .thumbnail() to Maintain Aspect Rations
from PIL import Image
with Image.open('/Users/datagy/Desktop/Original.png') as im:
print(im.size)
im.thumbnail((300, 150))
print(im.size)
# Returns:
# (930, 620)
# (225, 150)
Let’s see what the image now looks like:
Let’s see how we can now save the image using the .save()
method:
# Saving a Resized Image Using Pillow
from PIL import Image
with Image.open('/Users/datagy/Desktop/Original 2.png') as im:
im.thumbnail((300, 150))
im.save('/Users/datagy/Desktop/thumbnail.png')
How to Resize Multiple Images with Python
We can also use Python to resize images in bulk. This allows us to, for example, loop over all files in a directory and resize all the images in that directory.
Let’s combine what we’ve learned about the Pillow .thumbnail()
method and loop over files in a directory to return their resized versions:
# Resize all Images in a Directory
from PIL import Image
import os
directory = '/Users/datagy/Desktop/images'
for file in os.listdir(directory):
if file.endswith(('jpeg', 'png', 'jpg')):
filepath = os.path.join(directory, file)
outfile = os.path.join(directory, 'resized_'+file)
with Image.open(directory+'/'+file) as im:
im.thumbnail((300, 200))
im.save(outfile)
Let’s break down what we did here:
- We loaded the directory into a string. Change this to whatever folder is holding the images you want to resize.
- We then loop over each file in the
listdir()
method of the directory - If the file ends with a jpeg, png, or jpg, then we resize the image
- We do this by first getting the full path to the file and generating an outfile name for that file. The outfile simply prepends
'resized_'
to the front of the name of the file. - We then load the file using Pillow and use the thumbnail method to resize the image.
- Finally, we save the image using the
.save()
method.
Conclusion
In this tutorial, you learned how to resize images you Python’s Pillow library. You first learned how to open an image safely as a Pillow Image
object. Then, you learned how to resize images using the .resize()
and .thumbnail()
methods. Finally, you learned how to batch resize images using the os
library to loop over files in a directory.
Additional Resources
To learn more about related topics, check out the tutorials below:
Hi. You might use a free online service to resize image https://freetools.site/image-editors/resize